Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial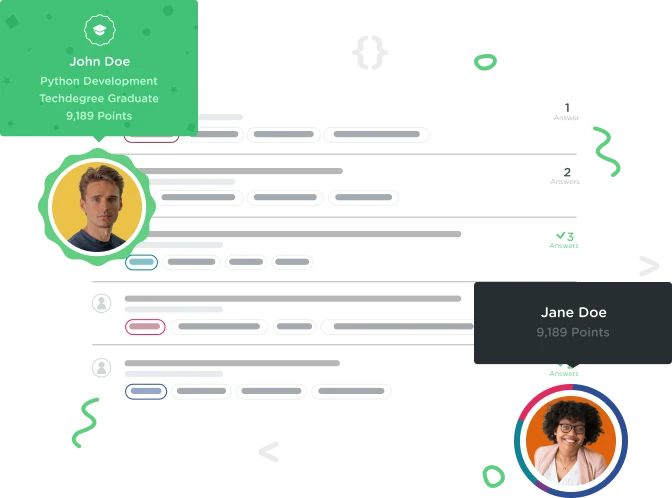

Guilherme Cunha
1,648 PointsProblems to solve the firts problem Unit Test
I'm try everything but the compiler error persist! :(
public class MainActivity extends AppCompatActivity implements MainActivityView {
TextView counter = "0";
Button button;
MainActivityPresenter presenter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
presenter = new MainActivityPresenter(this);
counter = (TextView) findViewById(R.id.textView);
button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
presenter.buttonCounterIsClicked(counter.getText().toString()));
}
});
}
@Override
public void counterIsClicked(String text) {
counter.setText(text);
}
}
public interface MainActivityView {
void counterIsClicked(String text);
}
public class MainActivityPresenter {
MainActivityView view;
public MainActivityPresenter(MainActivityView view){
this.view = view;
}
public void buttonCounterIsClicked(String text){
int count = Integer.parseInt(text);
view.counterIsClicked(Integer.toString(count + 1));
}
}
6 Answers

Seth Kroger
56,413 PointsYou pretty much had it down the first time. The only error was the line:
TextView counter = "0";
Which is a compiler error because you are assigning a String to an object that is not a String. Just leave it as TextView counter;
and it will be fine.

Guilherme Cunha
1,648 PointsI did the change, the problem persist...
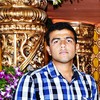
Sagar Suri
6,043 PointsCan you share what compiler error you are getting

Guilherme Cunha
1,648 PointsBummer! There is a compiler error. Please click on preview to view your syntax errors!
public class MainActivityPresenter {
Context context;
public MainActivityPresenter(Context context){ this.context = context; }
public void buttonCounterIsClicked(String text){ int count = Integer.parseInt(text); context.counterIsClicked(Integer.toString(count + 1)); } }
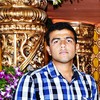
Sagar Suri
6,043 PointsInstead of this:
public MainActivityPresenter(MainActivityView view){ this.view = view; }
Replace with the following code in your MainActivityPresenter.java:
public MainActivityPresenter(MainActivity view){ this.view = view; }

Seth Kroger
56,413 PointsBut the entire point of the View/Presenter pattern is the is to abstract away the activity and context so it can be replaced with a mock implementation in the unit tests.
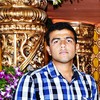
Sagar Suri
6,043 PointsInstead of this:
MainActivityView view;
public MainActivityPresenter(MainActivityView view){
this.view = view;
}
Write like this:
Context context;
public MainActivityPresenter(Context context){ this.context = context; }
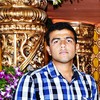
Sagar Suri
6,043 PointsSee what the actual problem is..in your MainActivity.java you have passed the context of MainActivity to MainActivityPresenter with the following code: presenter = new MainActivityPresenter(this);
But in your MainActivityPresenter.java you are trying to call the abstract of the MainActivityView through the MainActvity context. Declare the counterIsClicked() inside MainActivity or figure some other solution.