Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial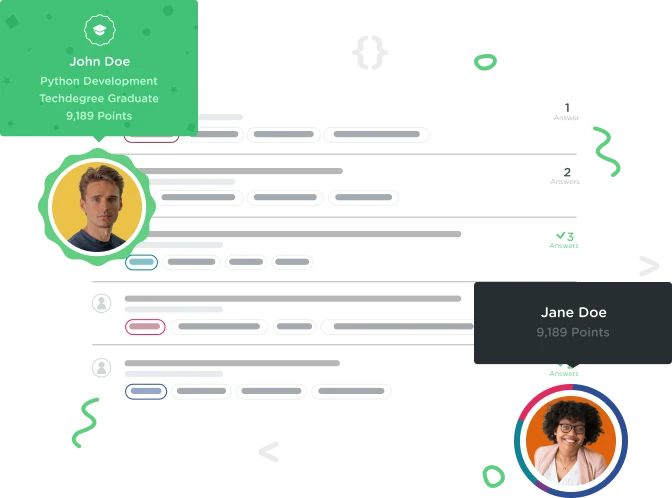

Amy Shah
25,337 Pointsproblems understanding the question
Instantiate an instance of the Context class within a using statement
I dont know if this begins in the top of the page with the other using statements
Use the Include operator to eagerly load the course's related teacher entity
does this go in the area where the green comment is ?
Use the SingleOrDefault LINQ operator to filter the available courses to the course whose Id property equals the passed in id parameter value
can anyone give an example of they mean by this last statement. I dont understand how to interpret
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
[Required, StringLength(200)]
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
return null;
using(var context = new Context())
{
return context.Courses.Where(o => o.Teachers.Equals(lastName)).ToList();
}
}
}
}
4 Answers
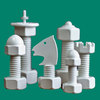
Steven Parker
231,533 PointsRemember, there are two kinds of "using statements".
Look in Repository.cs. The top 3 lines are one type, but on line 11 you will see an example of the other type. It's that second type that the challenge is talking about.
And you can use SingleOrDefault similarly to Where, by giving it a function parameter that filters the enumerable using some condition. This might be a good place for a lambda expression. There's an example of a Where with a lambda on line 25.

Gavin Schilling
37,904 Pointsusing System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses
.OrderBy(c => c.Teacher.LastName)
.ThenBy(c => c.Teacher.FirstName)
.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
return context.Courses
.Where(c => c.Teacher.LastName == lastName)
.ToList();
}
}
public static Course GetCourse(int id)
{
using (var context = new Context())
{
return context.Courses
.Include("Teacher")
.SingleOrDefault(c => c.Id == id);
}
}
}
}
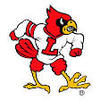
robert j bowman, jr
25,128 Pointslooking for an answer to this one
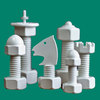
Steven Parker
231,533 PointsIf the hints given to Amy don't address your issue, you might start your own question. Be sure to include your properly formatted code and a link the course page (both are usually done for you if you use the "get help" button).

Daniel Eshetie
17,657 Pointspublic static Course GetCourse(int id) { using (var context = new Context()) { return context.Courses .Include( "Teacher") .SingleOrDefault(c => c.Id == id); } }

Amy Shah
25,337 PointsThanks for all replies. Appreciated it.
Daniel Eshetie
17,657 PointsDaniel Eshetie
17,657 Pointsusing System.Collections.Generic; using System.Data.Entity; using System.Linq;
namespace Treehouse.CodeChallenges { public static class Repository { public static List<Course> GetCourses() { using (var context = new Context()) { return context.Courses .OrderBy(c => c.Teacher.LastName) .ThenBy(c => c.Teacher.FirstName) .ToList(); } }
}