Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial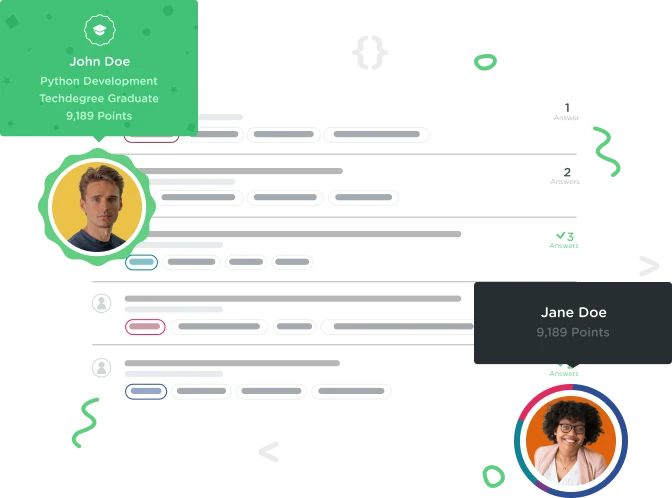
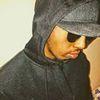
ibrahim Warsame
7,803 PointsProblems with accessing fields
Here is my code:
The code inside the StudentResults.java file
public class StudentResults {
public static String Full_Name;
private String Exam_Name;
private int Exam_Score;
private int Exam_Grade;
StudentResults(String fullName, String examName, int score, int grade) {
Full_name = "Ibrahim";
Exam_Name = "Matte_Tentamen";
Exam_Score = "100%";
Exam_Grade = "6";
}
}
The code inside the example.java file
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("Hi my first name is ibrahim, and i love programming in java");
StudentResults sIbrahim = new StudentResults();
System.out.println("Thise are the default values of the StudentResults object, %s", sIbrahim.Full_Name);
}
}
Here is what i get when i try to run the code inside the Example.java file.
Example.java:7: error: cannot find symbol
StudentResults sIbrahim = new StudentResults();
^
symbol: class StudentResults
location: class Example
Example.java:7: error: cannot find symbol
StudentResults sIbrahim = new StudentResults();
^
symbol: class StudentResults
location: class Example
2 errors
1 Answer
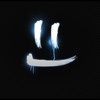
Grigorij Schleifer
10,365 PointsHi ibrahim,
I see some errors:
public class StudentResults {
public static String Full_Name; // variable names should start with lower case characters
private String Exam_Name;
private int Exam_Score;
private int Exam_Grade;
// dont forget to add a "public" access level modifier
// constructors are public and have no return type (no void too)
StudentResults(String fullName, String examName, int score, int grade) { //public StudentResults (....)
Full_name = "Ibrahim"; // change it to Full_name = fullName;
Exam_Name = "Matte_Tentamen"; // change it to Exam_Name = examName
Exam_Score = "100%"; // Exam_Score =score //
Exam_Grade = "6"; // Exam_Score = grade";
}
}
When you create the object of the StudentResults inside Example you will need to define the information of your student as constructor parameters and not inside the construcktor:
StudentResults sIbrahim = new StudentResults("Ibrahim", "Matte_Tentamen", 100, 6);
So inside Example class the code could look like this:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("Hi my first name is ibrahim, and i love programming in java");
StudentResults sIbrahim = new StudentResults("Ibrahim", "Matte_Tentamen", 100, 6);
// you providing constructor parameters when you create the object
// so different objects can have different parameters
// same as different students with diferent informations and grades and names ....
System.out.printf("Thise are the default values of the StudentResults object, %s", sIbrahim.Full_Name);
// changed println to print to use the string formatter %s
}
}
Let me know if this does the trick :)
Grigorij
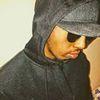
ibrahim Warsame
7,803 Pointsthis helped me alot. Thanks man :)
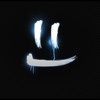
Grigorij Schleifer
10,365 PointsKeep on coding :)
See you in the forum
Grigorij
ibrahim Warsame
7,803 Pointsibrahim Warsame
7,803 PointsI'm trying to acess the fields that i created inside the StudentResults class. I'm new to this programming language so please tray to fix any bug that i made,. Thanks for advance.