Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial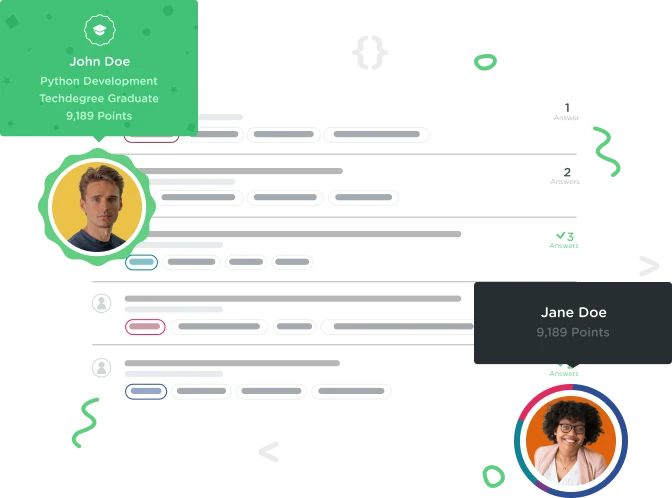
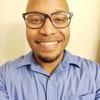
Anthony Smith
9,897 PointsProblems with my code in the Conditional Statements Challenge
I've completed the challenge (somewhat) and my code works and runs but I was unable to get any kind of if else statements to work so i resorted to just using if statements. Take a look and let me know how I can refactor this to be correct. I aplogize for the sloppy code, I should probably just start over and rewrite the whole thing but ive been stuck on this for 3 hours.
let correctAnswers = 0;
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
const correctOne = 'JavaScript';
const correctTwo = 'Muskegon';
const correctThree = 'Fishing';
const correctFour = 'Stacey';
const correctFive = 'Pizza';
// 2. Store the rank of a player
const rankOne = 'Gold';
const rankTwo = 'Bronze';
const rankThree = 'Silver';
const noRank = 'Sorry, you did not rank';
// 3. Select the <main> HTML element
// document.querySelector('main').innerHTML = `You got ${correctAnswers} out of 5 questions correct.
// Crown earned: ${}.`;
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const questionOne = prompt('Which programming language do you like the most?');
const questionTwo = prompt('What city did you grow up in?');
const questionThree = prompt('What is your favorite hobby?');
const questionFour = prompt('Who is your wife?');
const questionFive = prompt('Whats your favorite food?');
if (questionOne === correctOne) {
correctAnswers += 1;
document.querySelector('main').innerHTML = `<h2>You got ${correctAnswers} out of 5 questions correct.
// Crown earned: ${rankTwo}.</h2>`;
}
if (questionTwo === correctTwo) {
correctAnswers += 1;
document.querySelector('main').innerHTML = `<h2>You got ${correctAnswers} out of 5 questions correct.
// Crown earned: ${rankTwo}.</h2>`;
}
if (questionThree === correctThree) {
correctAnswers += 1;
document.querySelector('main').innerHTML = `<h2>You got ${correctAnswers} out of 5 questions correct.
// Crown earned: ${rankTwo}.</h2>`;
}
if (questionFour === correctFour) {
correctAnswers += 1;
document.querySelector('main').innerHTML = `<h2>You got ${correctAnswers} out of 5 questions correct.
// Crown earned: ${rankThree}.</h2>`;
}
if (questionFive === correctFive) {
correctAnswers += 1;
document.querySelector('main').innerHTML = `<h2>You got ${correctAnswers} out of 5 questions correct.
// Crown earned: ${rankThree}.</h2>`;
}
console.log(correctAnswers);
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
// 6. Output results to the <main> element
1 Answer

Cornelius Hager
5,659 PointsYou are on the right track. The multiple if
statements are actually correct way to check the answers to the questions. But the code is running from top to bottom. So you have to evaluate the score diferently.
- Remove all the
document.querySelector('main').innerHTML =...
from your if statements. This should already give you a correct console.log with the score you have. - Now you can work on the part of the code that returns the right result (score is not the result)
- Under List #5 you can create your
if else
statements to check the score and calculate the correct result. Here is a hint:
if (correctAnswers < 2) {
rank = "No crown"
} else if (correctAnswers === 1 || correctAnswers === 2) {
rank = "Bronze"
}...
- finally under #6 you can then write the final result to the HTML via your
document.querySelector('main').innerHTML =...