Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial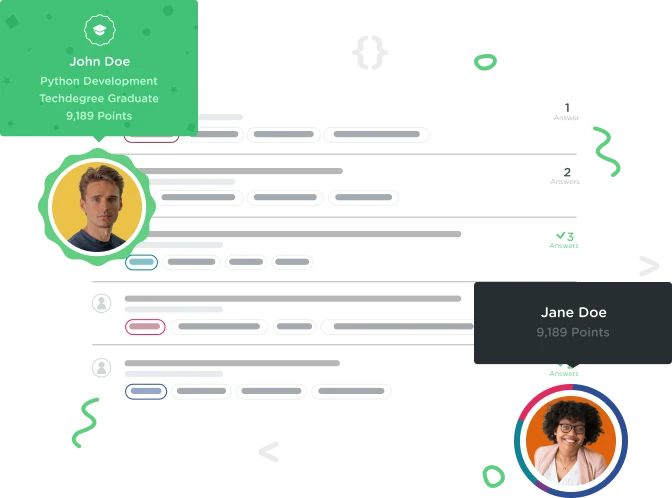
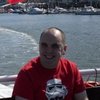
Sean Flanagan
33,235 PointsProblems with the var keyword
Andrew mentioned problematic or unexpected behaviours from using the var keyword. Exactly what kind of behaviours occurred?
3 Answers

Samuel Ferree
31,722 Pointsvariables defined with the var keyword are function level scoped. meaning they are defined once, for the entirety of your function, which doesn't play well with loops and lamdas imagine creating buttons with something like this
for(var i = 0; i < people.length; i = i + 1)
{
var person = people[i];
var button = createButton(person);
getList().addElement(button);
};
you might think that this would create a button for each person, one with each person's name. But since the var keyword scopes the button variable within the entire function... the button variable refers to one and only one button, and the person variable refers to one and only one person, both of which keep getting overidden, causing problems.
if you had declared the button variable with let instead of var You would see the intended result, since let has block level scoping. The person and button variables would be created anew each run of the loop.
var x = 1
let y = 2
{
var x = 3
let y = 4
console.log(x); // 3
console.log(y); // 4
}
console.log(x) // 3
console.log(y) // 2
In short, use let and const unless for some very special and very well understood reason, you need a global function variable, then and only then should you use var.
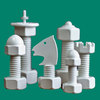
Steven Parker
231,007 PointsThe "var" keyword was easier to make mistakes with. You could accidentally re-declare variables without realizing it, or create shared globals in event handlers where you intended to provide unique values. Using "let" helps prevent these kinds of errors, and "const" goes a step further by insuring that a value that isn't intended to change never does.
I still use "var" for declaring things in global scope, as a way of indicating just that. But "let" is great for keeping things out of global scope that don't belong there.
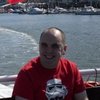
Sean Flanagan
33,235 PointsThanks guys!