Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial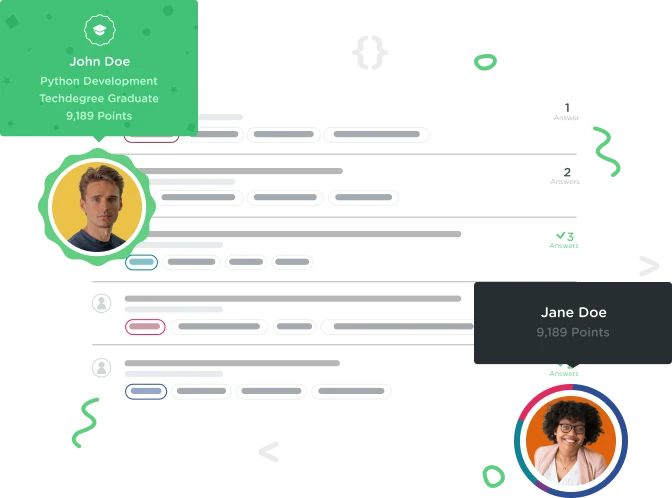
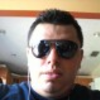
Eddie Flores
9,110 PointsProblems within the percent_letter.py tutorial.
I get to this point, and I'm doing okay. I'm familiar with Python, and I decide that I want to test the limits of this code.
user_string = input("What's your word? ")
user_num = input("What's your number? ")
try:
our_num = int(user_num)
except:
our_num = float(user_num)
if not '.' in user_num:
print(user_string[our_num])
else:
ratio = round(len(user_string)*our_num)
print(user_string[ratio])
I get back
treehouse:~/workspace$ python percent_letter.py
What's your word? Thunderbolt
What's your number? 5.3
Traceback (most recent call last):
File "percent_letter.py", line 13, in <module>
print(user_string[ratio])
IndexError: string index out of range
According to the tutorial, the round method is supposed to round to the nearest number, and the len will count the characters. It doesn't seem to work. I also tried the 2.7 version of this code on eclipse and I get this:
What's your word? Thunderbolt
What's your number? 5.4
Traceback (most recent call last):
File "/Users/testuser/Documents/workspace/test/src/pyFirst.py", line 13, in <module>
print(user_string[ratio])
TypeError: string indices must be integers, not float
From what I understand in the tutorial, no matter what number (within range) I put in there, it should round down to the nearest int and then execute the code that way. Otherwise, it will take it as a percentage like in the example he makes with the .75. Am I mistaken, or is there something wrong in the logic here?
2 Answers
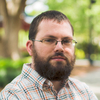
Kenneth Love
Treehouse Guest Teacher5.3
would be 530% of your string, so, yeah, that index doesn't exist. Ideally you'd throw an exception if someone gives a float that's bigger than 1.0
. Or, if the number is bigger than 1.0
, just loop through the string until you exhaust the part that's bigger than 100%.

Jason Anello
Courses Plus Student 94,610 PointsHi Eddie,
The way the code works is that if you enter an integer then the if
block is going to execute and that integer will be treated as in index into the string. So in your case you could enter any integer from 0 to 10 inclusive for "Thunderbolt"
If you enter a float, any float including 5.3, then the else
block will be executed and the number will be treated as a percentage of the way from the beginning. 5.3 is treated as 530% from the beginning which takes you way past the end to an invalid index.
11 * 5.3 = 58.3 and when you round that you get 58. You're trying to access the character at index 58 which is out of range.
So any float you enter needs to be between 0.0 and 1.0 inclusive. You can't go beyond 100% if you want to stay within range.
Actually, I think you need to stay below around .95 if using the round
function because beyond that it will round up to index 11 which is out of range.
Example: 11 * .98 = 10.78 which would round up to 11 and be just outside of the proper range. So I think with the code as written you can't quite go up to 100%