Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial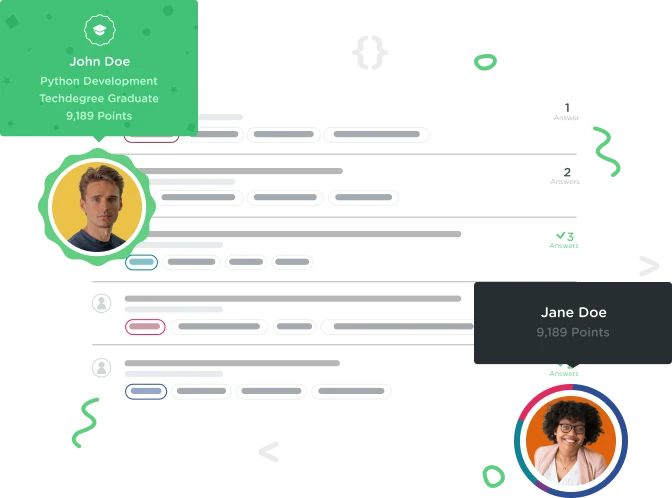

Cody James
2,387 PointsProcess is terminated due to StackOverflowException. C#
Hello, I have recently been messing around in C# and wanted to try out some of the new things I have learned. So I started to mess around with Accessors and every time I return the value of it I have been receiving "StackOverflowException". I'm trying to convert seconds to milliseconds as shown below.
namespace RPGConsole_Unamed
{
class Program
{
static void Main(string[] args)
{
Console.ForegroundColor = ConsoleColor.Blue;
GameText.SetSpeed(1);
GameText.Write(text: @"Hello World!!");
GameText.Write("Done");
Console.ReadKey();
}
}
class GameText
{
private static int _textspeed {
get
{
return _textspeed;
}
set
{
_textspeed = value*1000;
}
}
public static void SetSpeed(int seconds) => _textspeed=seconds ;
public static void Write(string text) {
for (int i =0; i<text.Length; i++) {
Thread.Sleep(_textspeed);
Console.Write(text.Substring(i, 1));
}
Console.WriteLine("");
}
}
}
Thanks for Reading!
2 Answers
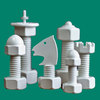
Steven Parker
230,274 PointsYou have a property that is referencing itself in its getter and setter.
So when you call the function that sets the property, the setter has to call the setter which calls the setter which calls the setter ... you get the idea. So the system quickly runs out of stack space.
Normally, you'd have a private field (with a name that starts with underscore) that is managed by a public property (with a non-underscore name). Then setting the property would not cause recursion. Also, you wouldn't need a setter method, you can just set the property directly.
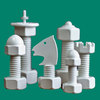
Steven Parker
230,274 PointsI see one possible issue.
Even with the code unformatted, this stood out for me at first glance:
public static void SetSpeed(int seconds) => seconds = _textspeed;
I'm guessing that the terms on each side of the assignment are reversed.

Cody James
2,387 PointsEven after fixing that mistake I'm still getting the error. I'm not to sure what I'm doing wrong here. I'm using visual studio 2017 and it's states the exception is on this line: C# _textspeed = value*1000;
Steven Parker
230,274 PointsSteven Parker
230,274 PointsTo make your posted code readable, use the formatting instructions in the Markdown Cheatsheet pop-up as found below the "Add an Answer" area.