Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial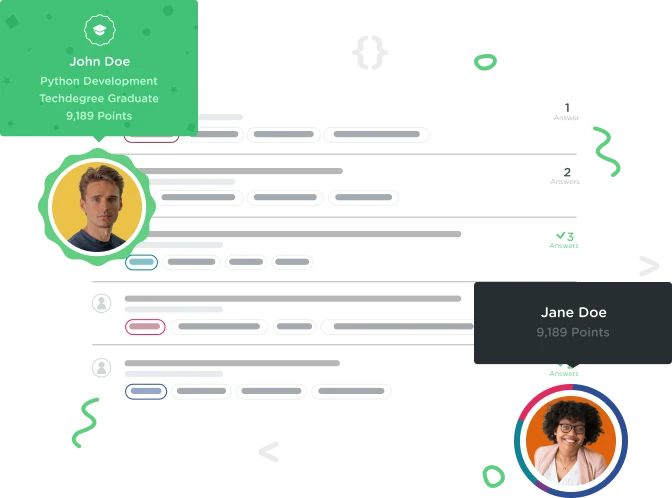

Unsubscribed User
3,309 PointsProcs & Lambdas Extra Credit: How to write your class without using a class variable?
Assuming I understood what was being asked for in the Extra Credit...
When I needed to call my proc from another method, I made an instance variable and kept getting no output. Since it works with class variables, this is a scope problem - is that correct? My code with an instance variable:
class ExtraCredit
def pass_a_proc(a_proc)
@my_proc = a_proc
end
def call_a_proc(n)
@my_proc.call n
end
end
sqr = ExtraCredit.new
sqr.pass_a_proc(proc {|x| x ** 2})
sqr.call_a_proc 4 # =>
Outputs nothing, but I checked and @my_proc.nil? == false
and @my_proc.respond_to? "call" == true
, inside both methods.
My code using class variables:
class ExtraCredit
def pass_a_proc(a_proc)
@@my_proc = a_proc
end
def call_a_proc(n)
@@my_proc.call n
end
end
sqr = ExtraCredit.new
sqr.pass_a_proc(proc {|x| x ** 2})
p sqr.call_a_proc 4 # => 25
p sqr.call_a_proc 7 # => 49
It feels clunky to have to use a class variable. Anyone know what the question is asking for? Can it be done with instance variables?
2 Answers

Unsubscribed User
3,309 PointsTo answer my own question:
Class instance variables are available only to class methods and not instance methods. Class variables are avilable to both class methods and instance methods.
That being said, http://stackoverflow.com/questions/3787154/why-should-class-variables-be-avoided-in-ruby/3787327#3787327 This in mind, I re-wrote my code to use class methods with a class instance variable:
class ExtraCredit
def self.pass_a_proc(a_proc)
@my_proc = a_proc
end
def self.call_a_proc(n)
@my_proc.call n
end
end
ExtraCredit.pass_a_proc(proc {|x| x ** 2})
p ExtraCredit.call_a_proc 7 # => 49
p ExtraCredit.call_a_proc 6 # => 36
I welcome any comments.

markd2
5,253 PointsThis is my code using the ampersand argument prefix to indicate that the method is expecting a proc. It may solve the scope issue above??
class ExtraCredit <br>
def saves_proc(&aproc) <br>
x = aproc <br>
end <br>
def calls_proc(&a) <br>
saves_proc(&a).call <br>
end <br>
end <br>
x = ExtraCredit.new <br>
a = Proc.new { puts "Hello world!" } <br>
x.calls_proc(&a) <br>
</p>