Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial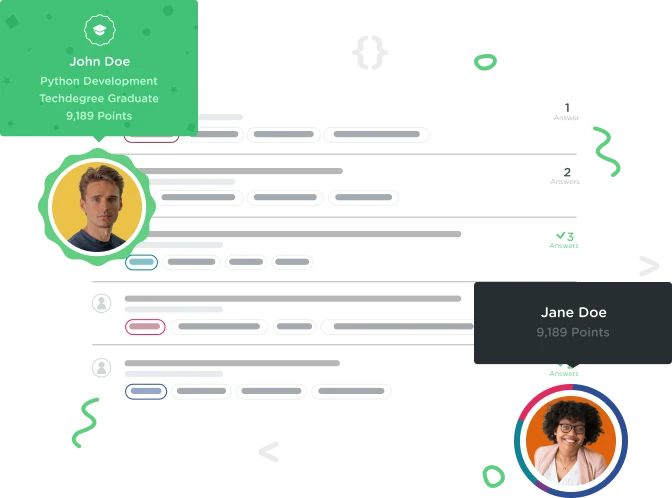
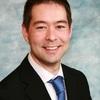
Mark Chesney
11,747 Pointsproduct_sales, reduce, total, prices
This is strange. It gives a non-descriptive "Bummer: Try again!"
But in my console, this completely works. In fact I've added:
if product_sum != (6.99*5) + (2.94*15) + (156.99*2) + (99.99*4) + (1.82*102):
raise ValueError("sum is incorrect")
Can anyone pinpoint the error here?
from operator import add
from functools import reduce
prices = [
(6.99, 5),
(2.94, 15),
(156.99, 2),
(99.99, 4),
(1.82, 102)
]
def product_sales(tuple_value):
price, units = tuple_value
return price * units
total = list(map(product_sales, prices))
product_sum = reduce(add, zzz)
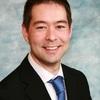
Mark Chesney
11,747 Pointsthank you Sandeep!
1 Answer
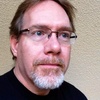
Chris Freeman
Treehouse Moderator 68,458 PointsYou are very close! You need to combine the last two lines into a single statement.
- rename
product_sum
to "total", and - replace the "zzz" with the
map(product_sales, prices)
from the previous line
Post back if you need more help. Good Luck!!
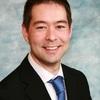
Mark Chesney
11,747 Pointsthank you Chris! turns out either of the two lines will work.
total = reduce(add, list(map(product_sales, prices)))
total = reduce(add, map(product_sales, prices))
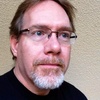
Chris Freeman
Treehouse Moderator 68,458 PointsRealize that the two lines are basically the same as reduce
takes an iterable as the second argument. map()
produces a generator which offers up one item at a time, and list(map())
simply unrolls the generator into a complete list. The unrolling is not necessary in this case.
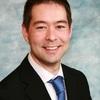
Mark Chesney
11,747 PointsI see. I have this from Data Science From Scratch:
A generator is something you can iterate over (usually using
for
) but whose values are produced only as needed (lazily)
Is there a Python Treehouse video or course that explains generators well? Thanks in advance!
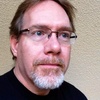
Chris Freeman
Treehouse Moderator 68,458 PointsI donβt know of a specific course. Kenneth mentions them in this video
Here is an outside reference on generator.
Sandeep Kumar
3,162 PointsSandeep Kumar
3,162 Points` from operator import add from functools import reduce
prices = [ (6.99, 5), (2.94, 15), (156.99, 2), (99.99, 4), (1.82, 102) ]
def product_sales(tuple_value): price, units = tuple_value return price * units
total = reduce(add,map(product_sales, prices)) ` Here in order to get total -
Reduce() - works in a way that it requires two values to work upon. If you see the map(product_sales,prices) part this r maps the prices list with the function product_sales and every iteration the product is returned so every iteration map returns a value. So on first iteration when map returns a value, reduce waits for the second value.
On second iteration, map returns another value - now when reduce() sees the second returned value it sums up both the values using the 'add' function - which also takes two inputs which is supplied by reduce from the map return values.
When add runs it add both those returned values and return one value. Now reduce has this value. Got it!. Now on third iteration of map, it returns a value again and now reduce already had the value returned from the add() function and now it gets the new value from map() , so again add runs and return another value which is the sum of old sum value with newly returned map value.
This process runs until all values are finished in map which contains iterable.
Hope this helped you. :)