Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial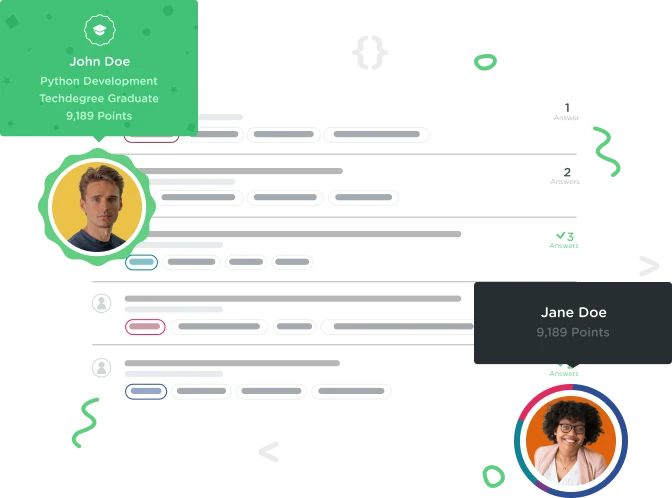

George Akinian
17,615 Pointsprofile page does not load profile information.
profile file
var EventEmitter = require("events").EventEmitter;
var http = require("http");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
rendere file:
var fs = require("fs");
function mergeValues(values, content) {
//Cycle over the keys
for(var key in values) {
//Replace all {{key}} with the value from the values object
content = content.replace("{{" + key + "}}", values[key]);
}
//return merged content
return content;
}
function view(templateName, values, reponse) {
//Read from the template file
var fileContents = fs.readFileSync('./views/' + templateName + '.html', {encoding: "utf8"});
//Insert values in to the content
fileContents = mergeValues(values, fileContents);
//Write out the contents to the response
reponse.write(fileContents);
}
module.exports.view = view;
router file
var Profile = require("./profile.js");
var renderer = require("./renderer.js");
//Handle HTTP route GET / and POST / i.e. Home
function home(request, response) {
//if url == "/" && GET
if(request.url === "/") {
//show search
response.writeHead(200, {'Content-Type': 'text/plain'});
renderer.view("header", {}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
}
//if url == "/" && POST
//redirect to /:username
}
//Handle HTTP route GET /:username i.e. /chalkers
function user(request, response) {
//if url == "/...."
var username = request.url.replace("/", "");
if(username.length > 0) {
response.writeHead(200, {'Content-Type': 'text/plain'});
renderer.view("header", {}, response);
//get json from Treehouse
var studentProfile = new Profile(username);
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
//Store the values which we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.badges.length,
javascriptPoints: profileJSON.points.JavaScript
}
//Simple response
renderer.view("profile", values, response);
renderer.view("footer", {}, response);
response.end();
});
//on "error"
studentProfile.on("error", function(error){
//show error
renderer.view("error", {errorMessage: error.message}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
});
}
}
module.exports.home = home;
module.exports.user = user;
I have a console.log error in a workspace as follows:
treehouse:~/workspace$ node app.js
Server running at http://<workspace-url>/
events.js:85
throw er; // Unhandled 'error' event
^
Error: connect ETIMEDOUT
at exports._errnoException (util.js:746:11)
at TCPConnectWrap.afterConnect [as oncomplete] (net.js:1010:19)
=============================
Hi Andrew Chalkley, I'v isolated the timeout to http.get at the following url: http://teamtreehouse.com/paulwagner.json
When i browse to it directly with my browser i have no problem getting to it. When i substitute http://google.com in that same http.get call it returns no problem. I suspect there maybe something blocking the communication between your app server and your api server.
3 Answers
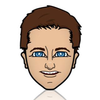
Colin Stodd
6,257 Points+1
My page actually loads the error page and not the profile page. Im assuming its because Im getting a 404 error.
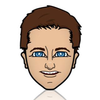
Colin Stodd
6,257 PointsBut Paul to answer your question. You might have to add an "s" to any place where you have http://www.teamtreehouse.com"
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
Treehouse added the "s" security recently. Not sure why they haven't upgraded the videos to reflect this.
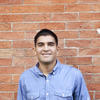
Wilson Usman
35,206 PointsAdding the s to http doesn't solve my problem. I really wish we could find the solution to this... :/
I also want to point out that I'm using the project download files. Even the last folder won't work, all it executes is the username back without any styling.
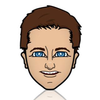
Colin Stodd
6,257 PointsSorry I can't find how to edit my posts; but I meant (above) the "s" as in "http(s)", so anywhere http://www.teamtreehouse.com is included in your code, you need to change it to https://www.teamtreehouse.com