Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial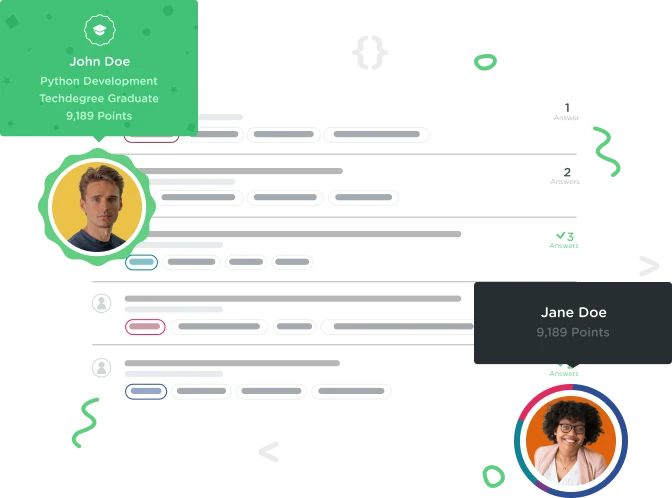
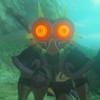
tomasvukasovic
24,022 PointsProfiles have been moved, Course needs to be updated
If you want to do this course on May 2016, you may come across some problems such as trying to get the profiles. This profiles have been moved by treehouse to https protocol, SO I added my solution to it, so you can keep working on node
3 Answers
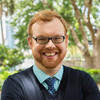
Andrew Chalkley
Treehouse Guest TeacherI just launched the workspace associated with this video:
var EventEmitter = require("events").EventEmitter;
var https = require("https");
var http = require("http");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
var profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
And that's the code in the profile.js file. Did you start this course a while ago and come back to it?
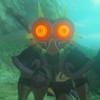
tomasvukasovic
24,022 PointsSo the way to fix this is by updating the profile.js
file to this: (I imported https
, updated the urls AND modified the profileEmitter when it catches and error take a look at the response.statusCode
)
var EventEmitter = require("events").EventEmitter;
var http = require("https");
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
// Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + response.statusCode + ")"));
}
// Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
// Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
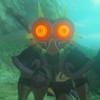
tomasvukasovic
24,022 Pointstomasvukasovic
24,022 PointsYes! In fact I did all Node.JS course and thought about refreshing some concepts so I tackled the course again
Andrew Chalkley
Treehouse Guest TeacherAndrew Chalkley
Treehouse Guest TeacherThat explains it! Yeah, when you fork a workspace you copy the code at that point in time. All new students will get the updated code.
tomasvukasovic
24,022 Pointstomasvukasovic
24,022 PointsAndrew Chalkley THANKS! Yeah im checking the profile JS again to understand a little bit more the code, thanks Andrew.