Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial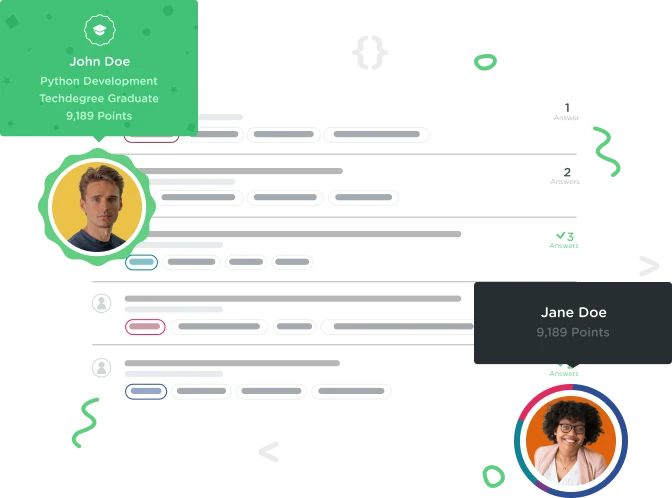

Justin Esders
9,154 PointsProgram
So I have a program I am supposed to complete that has to show a random card 1-52.
Somehow I have to do two loops that are
- for the suit
- for the card 1-13
Basically either how do i accomplish building this string into the image of a card or can someone point me in the direction of building an image from a string.
I have searched but i think that i am searching incorrectly.
Thanks
7 Answers
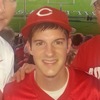
Scott Kipfer
10,348 PointsThere are plenty of ways to accomplish this.
This is the first thing that popped into my head.
<?
$suit = array("hearts", "diamonds", "clubs", "spades");
$card = array("ace","two", "three","four","five","six","seven","eight","nine","ten","jack","queen","king");
$randsuit = rand(0,3);
$randcard = rand(0,12);
echo ("The random card is the ".$card[$randcard]." of ".$suit[$randsuit]."!");
echo ('<img src="img/'.$card[$randcard].'of'.$suit[$randsuit].'.png">');
The trick here to get the string of the suit and the string of the card and concatenate them together. There is a video on this in the PHP section. here
Now make sure to name all of your images correctly (i.e. aceofheards.png, jackofspades.png)
If you have any questions let me know.

Justin Esders
9,154 Pointsso theoretically i should be able to pull those from an images folder?
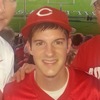
Scott Kipfer
10,348 Pointssure. the out put of the last echo for random numbers (0,0) is:
<img src="img/aceofhearts.png">
so if you have a folder named "img" with the file "aceofhearts.png" located in it it will show up just fine.
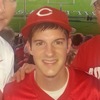
Scott Kipfer
10,348 Pointssorry did not format the code properly.
the output of the echo is is:
<img src="img/aceofhearts.png">

Justin Esders
9,154 PointsSo what about user input....If i wanted a number of cards that the user enters 1-52 how would i do that

Justin Esders
9,154 Pointsand all the cards have to be different that the user gets for example....the user enters 52 he gets the whole deck...if he enters 5 he gets 5 cards.... but all different....I tried a for loop with the user input but it doesnt seem to work
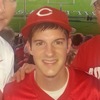
Scott Kipfer
10,348 PointsDoes this have to be strictly php?

Justin Esders
9,154 PointsYes and HTML I go to a different php page when the user enters their number.
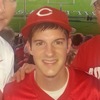
Scott Kipfer
10,348 PointsHere is something quick and dirty... I did not add user input .. I just hard coded a number... I figure you can handle adding the user input. If you have any questions let me know.
<?php // Card Class ------------------------------------------------------------------------------------------------
class Card {
// Card Suit (hearts,diamonds,spades, clubs)
public $card_suit = "Hearts";
// Card Type (Ace, Two, Three, .... King)
public $card_type = "Ace";
/* this constructor function uses random numbers to generate a new card */
function Card () {
$suit = array("Hearts", "Diamonds", "Clubs", "Spades");
$card = array("Ace","Two", "Three","Four","Five","Six","Seven","Eight","Nine","Ten","Jack","Queen","King");
$this->card_suit = $suit[rand(0,3)];
$this->card_type = $card[rand(0,12)];
}
/* This function takes in anrray of cards and checks to see if this card is already a part of it */
function isInArray ($arrayOfCards) {
if(count($arrayOfCards) != 0){
foreach ($arrayOfCards as $Card){
if($this->card_suit == $Card->card_suit && $this->card_type == $Card->card_type){
return true;
}
}
}
return false;
}
/* This function will generate an image tag with the format <img src="img/AceofHearts.png">
for example */
function generateImage(){
echo ('<img src="img/'.$this->card_type.'of'.$this->card_suit.'.png">');
}
/* THis function will pring out the card info */
function printCard() {
echo $this->card_type." of ".$this->card_suit;
}
}
// End of Card Class --------------------------------------------------------------------------------------
// Actual Program ------------------------------------------------------------------------------------------
// This Array will hold the users Cards
$usersCards = array();
/* Here is where you can insert the user input... instead of a hardcoded number (ie 5)
you could use the user input as a variable */
for ($i=0;$i<5;$i++){
$newCard = new Card();
while($newCard->isInArray($userCards)){
$newCard = new Card();
}
$userCards[] = $newCard;
}
//Output The Array of Users Cards.
echo "<h1> Your Cards are: <br></h1>";
foreach ($userCards as $userCard){
echo"The ";
$userCard->printCard();
$userCard->generateImage();
echo "<br>";
}
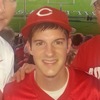
Scott Kipfer
10,348 PointsIf you wanted to you could make the Card Class a separate PHP file (i.e. - Card.php) and then include it in your main file (i.e. - include ("inc/Card.php"); . This might make things look a little cleaner.

Justin Esders
9,154 PointsIs there a simpler way....like i feel stupid asking you to keep helping and i appreciate it. can you somehow show me a simpler way say using and if else block with an array being generated as you go so there are no duplicate cards. does that make sense at all?
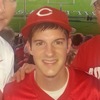
Scott Kipfer
10,348 PointsSure I could, but... What exactly is this for? School work? Why would you like it "simplified"? Is there something that you don't understand?

Justin Esders
9,154 PointsYea I just don't understand how to generate the array. I just want to know how to do it I can do it if I have the step by step process its clicking but just not enough yet. I feel like i either need point in right direction or an example of how to do this.
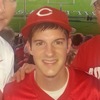
Scott Kipfer
10,348 PointsHave you ever used classes in php before?
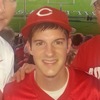
Scott Kipfer
10,348 PointsGreat, then I will try to explain it to you and if you still are having trouble, I will re-write it with out using classes.
So where the array of users cards gets generated is in this part
for ($i=0;$i<5;$i++){
$newCard = new Card();
while($newCard->isInArray($userCards)){
$newCard = new Card();
}
$userCards[] = $newCard;
}
I will try to break it down.
-
for loop - obviously you get this... loops through 5 times. If you wanted any number of cards (1-52) you would need to get in put from the user and pass it to a variable say $number_of_cards then your for loop would be structured like this:
for ($i=0;$i<$number_of_cards;$i++){ ....... }
-
$newCard = new Card(); - This creates a new instance of the class Card. Every time you create a new instance of a class you have the opportunity to run a constructor function. This is just the name of the class. The constructor function function Card () { $suit = array("Hearts", "Diamonds", "Clubs", "Spades"); $card = array("Ace","Two", "Three","Four","Five","Six","Seven","Eight","Nine","Ten","Jack","Queen","King");
$this->card_suit = $suit[rand(0,3)]; $this->card_type = $card[rand(0,12)];
}
The constructor function here generates a card by filling in the public variables from the class. You can access the public variables of a class by using $this->some_variable. This will look at variables of "this" particular instance.
while($newCard->isInTheArray($usersCards)) - This is where I make sure that the card has not already been drawn. I use the class function isInTheArray($somearray) that I defined in the class. This compares the new instance of the Card class against the array of Cards that have already been created. If the function returns true then the card is in the array and I create a new instance of the Card Class and check that against the array of usersCards. If the Card is not in the array then I know it hasn't been drawn yet.
$userCards[] = $newCard; - this line of code adds the newly created card to the existing array of the users Cards. $array[] =$something will always add $something to the end of $array.
Does this help at all? Sorry I am not very good at explaining things. I would recommend doing the PHP videos.
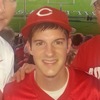
Scott Kipfer
10,348 Pointssorry it didn't format the constructor function very well.
function Card () {
$suit = array("Hearts", "Diamonds", "Clubs", "Spades"); $card = array("Ace","Two",
"Three","Four","Five","Six","Seven","Eight","Nine","Ten","Jack","Queen","King");
$this->card_suit = $suit[rand(0,3)];
$this->card_type = $card[rand(0,12)];
}

Justin Esders
9,154 Pointshmm not in php but i have in java c# and so on. I know the basic concept

Justin Esders
9,154 Pointswhat is the (->)? can i replace this with something else?

Justin Esders
9,154 Pointsdo you mind rewriting it without classes so i can see the difference. Sorry I dont mean to be a pain
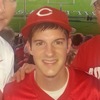
Scott Kipfer
10,348 PointsYeah give me a little time I will rewrite it.

Justin Esders
9,154 Pointsagain i appreciate the help and thanks a bunch

Justin Esders
9,154 Pointshow is that php thing going need anything from me?
Scott Kipfer
10,348 PointsScott Kipfer
10,348 PointsAs a future note: To get a faster response try and be a little more descriptive in the title of your post.