Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial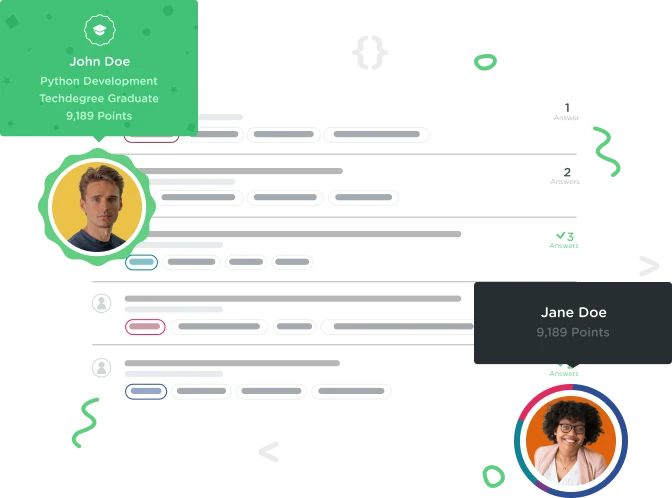

Georg Ekeberg
5,702 PointsProgram breaks when on of the promises are rejected. (Namespelling discrepancy issue.)
Since Wikipedia spells Anatoly's name with an I at the end, I get a 404 error on that specific promise. This gives me the following error:
Error at XMLHttpRequest.xhr.onload (promises.js:15)
How do I make sure that the program works, even if this happens?
4 Answers
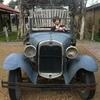
Brendan Moran
14,052 PointsUse Promise.allSettled(). The following code works, it's simply going to skip over the rejected promise:
function getWikiProfiles(data) {
const profiles = data.people.map(person => {
return getJSON(wikiUrl + person.name);
});
return Promise.allSettled(profiles);
}
function generateHTML(data) {
//console.log(data);
data.forEach(person => {
if (person.status === 'fulfilled') {
person = person.value;
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
}
});
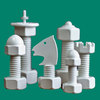
Steven Parker
231,275 PointsThis issue is due to two 3rd-party API's having a disagreement about spelling. Code that would handle this kind of thing in a generic way would be rather complex.
But if you don't mind applying a temporary fix for these specific cases, see the code posted by another student in reponse to this other question
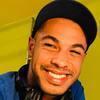
Murilo de Melo
14,456 PointsGreat solution. Tnx

Christian Jorgensen
8,352 PointsThank you:)
Sean Ball
10,501 PointsSean Ball
10,501 Pointswhere exactly is getWikiProfiles being called?
Mat T'Qartit
29,277 PointsMat T'Qartit
29,277 PointsI had the same problem but your code helped thanks alot