Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial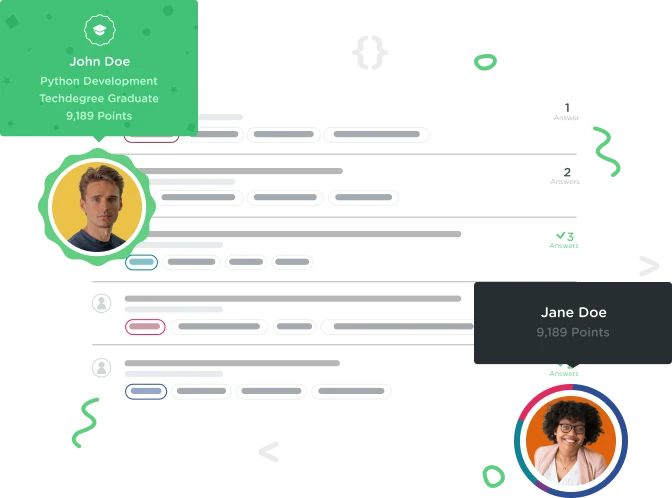
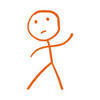
Vladimir Ulyanov
14,023 PointsProgram format best practice?
Hi everyone, I'm currently on the Python track and am loving the lessons so far.
I challenged myself to build the disemvowel program before watching this video, and it looks wildly different to what Kenneth has put together. I understand it's a different concept as Kenneth's program takes a set list of words already where as mine takes an input from the user.
That being said, my question lies more towards the formatting (not even sure that's the right word!) side of programming.
The code I have written uses functions and a while loop as going from previous videos this seems like a logical code format to use when creating an input loop that the user can control.
I was wondering if someone could take a look at what I built and give me some general pointers on if the way I have programmed this. Is it following good practice or are there areas I could improve on? I'm not too sure how much code should be held in the functions themselves and if I should leave as much coding out of the main loop as possible?
I basically want to try and learn good formatting habits early so I don't end up writing messy code in the future!
Code:
import os
def clear():
if os.name == "nt":
os.system("cls")
else:
os.system("clear")
def get_word():
while True:
try:
word = list(str(input("What word shall I disemvowel? ")).lower())
except ValueError:
print("I can only disemvowel words, you pleb.")
else:
return word
def disemvowel(any_word, letters):
for letter in letters:
while True:
try:
any_word.remove(letter)
continue
except ValueError:
break
print("Behold! Your disemvoweled word!")
print("".join(any_word).capitalize())
def restart():
restart = input("Type RESTART to disemvowel another word, type anything else to quit. ").lower()
if restart == "restart":
return 1
else:
return 0
def welcome():
print("I am the mighty disemvoweler!")
input("Press Enter to begin! ")
welcome()
while True:
clear()
disemvowel(get_word(), "aeiou")
if restart():
continue
else:
break
If this question is better suited to be asked somewhere else, please let me know, and thank you :)
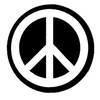
john larson
16,594 PointsJames, I love this approach you took here. So I ran it and started dissecting it to see how you did it. One thing I found was that since input always returns a string, if I type in 20 for a word it gives back 20 as a disemvoweled word. In this case there is no value error cause your checking for a string and you always get a string. Aside from that, I'm using your code as a lesson all in itself. Very nice :D I guess I should mention I'm referring to the get_word function.
1 Answer
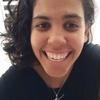
Rebeca Sarai
4,609 PointsHey man, about the functions, code them for make your life easy, that is, use to avoid code repetition. About the welcome() function since you put out of the loop it didn't need to be a function since there is no reuse. But these are silly things, you're doing great
ronakeogh
30 Pointsronakeogh
30 PointsThere is no need to have your str function in the get_word function, as any user input taken in through the input function automatically becomes a string, doing this will decrease your parentheses and therefore make your could more readable. This also means you can remove your try.
Also where are you getting the parameters for your functions from.