Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial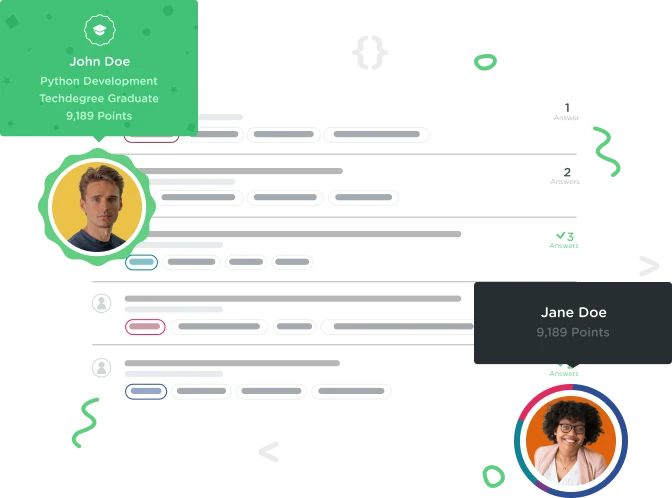
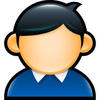
Daniel Hildreth
16,170 PointsProgram when ran does not return any birds
So I figured out why it didn't compile thanks to Steven, but now my list of birds is not returning in the mono program.exe. What am I doing wrong this time?
BirdSearchExtension.cs
using System.Collections.Generic;
using System.Linq;
namespace BirdWatcher
{
public class BirdSearch
{
public string CommonName { get; set; }
public List<string> Colors { get; set; }
public string Country { get; set; }
public string Size { get; set; }
public int Page { get; set; }
public int PageSize { get; set; }
}
public static class BirdSearchExtension
{
public static IEnumerable<Bird> Search(this IEnumerable<Bird> source, BirdSearch search)
{
return source.Where(s => search.CommonName == null || s.CommonName.Contains(search.CommonName))
.Where(s => search.Country == null || s.Habitats.Any(h => h.Country.Contains(search.Country)))
.Where(s => search.Size == null || s.Size == search.Size)
.Where(s => search.Colors.Any(c => c == s.PrimaryColor) ||
search.Colors.Any(c => c == s.SecondaryColor) ||
search.Colors.Join(s.TertiaryColors,
sc => sc,
tc => tc,
(sc, tc) => (sc)).Any())
.Skip(search.Page * search.PageSize)
.Take(search.PageSize);
}
}
}
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace BirdWatcher
{
public class Program
{
public static void Main(string[] args)
{
var searchParameters = new BirdSearch
{
Size = "Medium",
Country = "Unnited States",
Colors = new List<string> { "White", "Brown", "Black" },
Page = 0,
PageSize = 5
};
Console.WriteLine("Type any key to begin search");
var birds = BirdRepository.LoadBirds();
while(Console.ReadKey().KeyChar != 'q')
{
Console.WriteLine($"Page: {searchParameters.Page}");
birds.Search(searchParameters).ToList().ForEach(b =>
{
Console.WriteLine($"Common Name: {b.CommonName}");
});
searchParameters.Page++;
}
}
}
}
Console
treehouse:~/workspace$ mcs *.cs -out:Program.exe
treehouse:~/workspace$ mono Program.exe
Type any key to begin search
Page: 0
Page: 1
treehouse:~/workspace$
2 Answers

Roman Fincher
18,267 PointsThis will take a little digging to find out and I can't look into it for a little while. However, if you haven't already installed Visual Studio, now would really be a great time to do so. You need a debugger! If you're not familiar, check out: Debugging in Visual Studio Treehouse Course.
Basically, this will let you go through line by line to see which piece of the code is not returning what you expect. It will make it much so easier to correct issues like this.
At this point in the courses, I think it's probably in your best interest to start working in Visual Studio instead of Workspaces. You'll have a lot more tools at your disposal.

Emanuel Vargas
2,724 PointsI know this post is a year old, but for anyone running into a similar issue, the problem in this case is that you've misspelled "United States". You have two "n"s. The program is correct in returning 0 birds because there are no birds in the list with the country of "Unnited States". If no birds are being returned, make sure your search parameters are correct.
Daniel Hildreth
16,170 PointsDaniel Hildreth
16,170 PointsOk I'll try converting this project over to Visual Studio maybe tonight, and then go from there.