Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial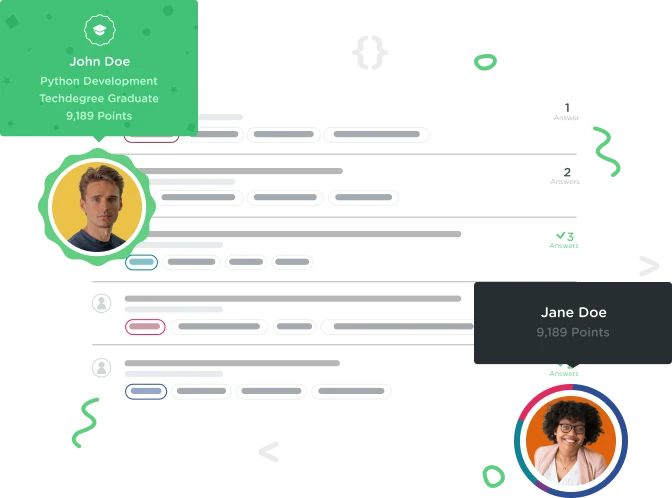

Hakim R
355 PointsProgram.cs(11,13): error CS0103: The name `input' does not exist in the current context
?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try
{
input = Console.ReadLine();
if (input == "quit")
{
string output = "Goodbye.";
}
else
{
string output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
catch(FormatException)
{
continue;
}
}
}
}
2 Answers

Jeff Wilton
16,646 PointsYou need to declare your variables before you set them to some value.
In this case, 'input' needs to be a string so we declare it like this:
string input = Console.ReadLine();
Next thing, when you declare a variable, it can now be used anywhere within the function it was declared in as indicated by curly braces { }. It can even be used in other functions or if/else blocks, etc, that are inside of the function the variable was declared in. Example:
try {
string outerVariable = "You can see me!";
if(1=1) {
Console.WriteLine(outerVariable); //prints "You can see me!"
}
}
However, a variable declared inside a function can not typically be seen Outside of that function. Example:
try {
if(1=1) {
string innerVariable = 'Uh oh!";
}
Console.WriteLine(innerVariable); //compiler error
}
So how do we fix this? If you want to access a variable Outside of a function where its value is set, then you can simply declare the variable in the outer function and set its value inside the inner function like this:
try {
string innerVariable = "";
if(1=1) {
innerVariable = "Hello World!";
}
Console.WriteLine(innerVariable); //prints "Hello World!"
}
The last problem with your code is that you are using the looping keyword 'continue' but you are not inside of a loop. That will throw a compiler error as well.
Having said all that, here is a working version of your code:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try
{
string input = Console.ReadLine();
string output = "";
if (input == "quit")
{
output = "Goodbye.";
}
else
{
output = "You entered " + input + ".";
}
Console.WriteLine(output);
}
catch(FormatException)
{
//continue; <- this line can be removed
}
}
}
}

Hakim R
355 PointsThanks a lot :)