Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial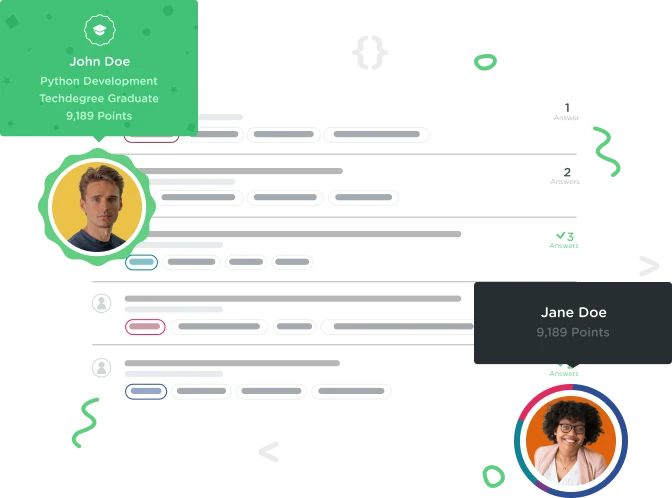

Camron Schwoegler
4,784 PointsProgramming a Background Image for iPhone 5
With the introduction of the iPhone 5 and iOS 6 SDK it seems that there is a new method to handling views due to the different display sizes. When adding a UIImageView programmatically and then using the iPhone (Retina 4-inch) Device in the iOS Simulator the image doesn't scale vertically and this results in a grey box at the bottom of the screen where the Predict button sits. This also causes the label text to sit lower in the crystal ball. When using Interface Builder one can set the size of the UIImageView such that its constraints force it to resize when using the different sized displays. Are there plans to address this with new videos or a written update with information on how to achieve this programmatically as well?
Thanks!
12 Answers
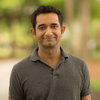
Amit Bijlani
Treehouse Guest TeacherSorry my solution was incomplete. Here's the complete answer:
To enable the app for the taller screen you have to first include an image named Default-568h@2x.png
Create a macro to detect the iPhone 5 (see blog post section "iPhone 5 compatibility" on details: http://blog.teamtreehouse.com/creating-a-iphone-app-bearded-part2 )
-
Create a taller version of the background image and then add this code to your
viewDidLoad
methodUIImage *image; if ( IS_IPHONE_5 ) image = [UIImage imageNamed:@"background-tall.png"]; else image = [UIImage imageNamed:@"background.png"]; self.imageView = [[UIImageView alloc] initWithFrame:self.view.bounds]; self.imageView.image = image; self.imageView.autoresizingMask = UIViewAutoresizingFlexibleHeight; self.imageView.contentMode = UIViewContentModeScaleAspectFill; [self.view insertSubview:self.imageView atIndex:0];
Here's the code if you are interested: http://treehouse-code-samples.s3.amazonaws.com/iPhoneIslands/CrystalBall-iPhone5.zip

Ernest Grzybowski
Treehouse Project ReviewerYes, it would be awesome if someone could answer this question. I've been having this same problem, just haven't gotten around to asking it yet. If @Amit reads this by any chance or any other employee, can you give us a timeline on how long it will take for the videos to be updated? There are a few things that have really messed with me (such as this) that could have been avoided. Since this is a paid service, I would just love to at least have a timeline on this, so I know what to expect and when.
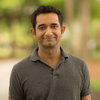
Amit Bijlani
Treehouse Guest TeacherThe latest version of Xcode introduces Autolayout which can be disabled to avoid these issues. See my blog post on how to disable it:
- http://blog.teamtreehouse.com/creating-a-fun-iphone-app-called-bearded-part-1
- or this image: http://blog.teamtreehouse.com/wp-content/uploads/2012/12/04-autolayout.png
To make your image view stretch vertically after disabling Autolayout you can add the following:
self.imageView.autoresizingMask = UIViewAutoresizingFlexibleHeight;
We will not be updating these videos since it's a feature that can be disabled. We will teach Autolayouts in the upcoming Blog Reader project.

Ernest Grzybowski
Treehouse Project ReviewerHmm... just a tip, maybe you guys can include that link in the description of your video? I didn't even know you guys had a blog with information that wasn't on this website. Hahaha. I appreciate pointing us in the right direction.

Camron Schwoegler
4,784 Points@Amit Any chance you tested this with the code from the Crystal Ball app firsthand? I've disabled Autolayout in Mainstoryboard.storyboard and have tried adding the above line of code to ViewController.m but I see no changes to the image when using the iPhone (Retina 4-inch) Device.

Ernest Grzybowski
Treehouse Project ReviewerWhere do you add:
self.imageView.autoresizingMask = UIViewAutoresizingFlexibleHeight;
I can't quite get that line of code to work. At least, it won't compile. What about you @Camron? I'm just trying to make sure my app background's will work seamlessly across all devices.

Camron Schwoegler
4,784 Points@Ernest I put it in the viewDidLoad method in ViewController.m. Mine compiles without errors but does not affect anything. I've also tried using the sizeToFit method on the imageView to no avail.

Ernest Grzybowski
Treehouse Project Reviewer@Camron
This is a chunk of my code. It won't compile. Any ideas? I get an error on the last line.
- (void)viewDidLoad
{
[super viewDidLoad];
UIImage *myImage = [UIImage imageNamed:@"background.png"];
UIImageView *myImageView = [[UIImageView alloc] initWithImage:myImage];
[self.view insertSubview:myImageView atIndex:0];
self.myImageView.autoresizingMask = UIViewAutoresizingFlexibleHeight;

Camron Schwoegler
4,784 Points@Ernest Since you've declared myImageView within the block viewDidLoad it is an instance variable and not a property. As a result you shouldn't try and reference it with the self. notation.
myImageView.autoresizingMask = UIViewAutoresizingFlexibleHeight;

Ernest Grzybowski
Treehouse Project ReviewerThat actually makes total sense. This whole property thing in Objective-C is a bit confusing. Also, the whole header/implementation files are also throwing me off as well.
Edit: Just added in the line of code (and it compiles) but I don't see any difference either.

Ernest Grzybowski
Treehouse Project ReviewerBeautiful. Thank you!

Ralph Ritter III
Courses Plus Student 476 PointsThanks for providing your revisions Amit, this was very helpful!
Paul Cisneros
1,332 PointsPaul Cisneros
1,332 PointsCould this be done for the animated images as well? An if statement?
Something like:
<pre> self.imageView.animationImages = animation;
if (IS_IPHONE_5) animation = [[UIImage imageNamed:@"ball_iphone5.png"], ... nil]; else animation = [[UIImage imageNamed:@"ball.png"], ... nil]; </pre>
Amit Bijlani
Treehouse Guest TeacherAmit Bijlani
Treehouse Guest TeacherSure, except you would have to assign your image view an array of
UIImage
objects.