Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial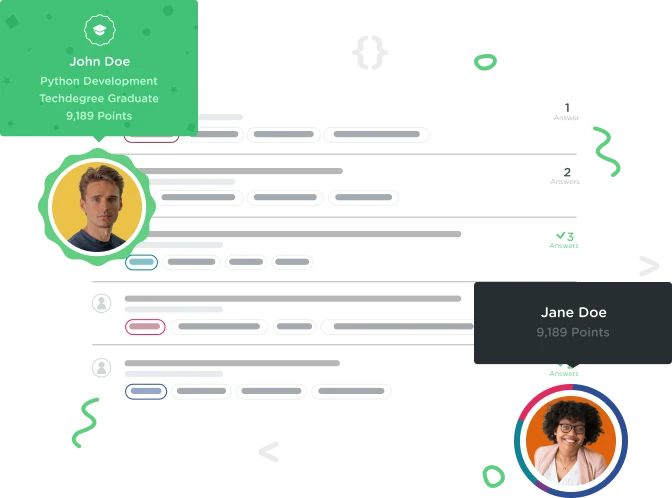

Johnathan Wenske
3,410 PointsProgramming Extra Credit
Hello! Under the Programming section of the Front End Development track there is an Extra Credit option at the bottom. I can't seem to figure out how to do it. Will someone help me. I only can do what the videos taught so I'm pretty useless right now.
Jim Hoskins , any suggestions on how to do this?? I'm so lost.
Write a program that loops through the numbers 1 through 100. Each number should be printed to the console, using console.log(). However, if the number is a multiple of 3, don't print the number, instead print the word "fizz". If the number is a multiple of 5, print "buzz" instead of the number. If it is a multiple of 3 and a multiple of 5, print "fizzbuzz" instead of the number.
Hint. Use loops and if/else statments. In javascript the % is the modulo, or remainder operator. a % b evaluates to the remainder of a divided by b. 11 % 3 is equal to 2.
Thank you!! Johnathan
9 Answers

Jorge Eduardo Garcia Celorio
10,112 PointsOk, before I move on, just a tiny remark. I missed the word "var" in the for loop in the previous post. We need to type
for(var i = 1; i<=100; i++){
console.log(i);
}
where var defines our index variable, which is i. Check it out, I also corrected it in the previous post.
So, we already have our numbers 1 to 100 printed to the console. Now, let's break into the fizz word which is the word that needs to be printed everytime we have a number that can be divided by 3. Here, we need a conditional.
The condition will be based on the following logic
if( our index, i, is a number that can be divided by 3){
console.log("fizz"); //and it will print to the console the word fizz
} else {
console.log(i); //since we don't have a number that can be divided by 3, just print the number.
}
The if condition needs to compare the value of i using the modulo operator '%'. If the modulo operation is 0, (i%3 == 0) it means that we have a number that can be divided by 3!
So the code would be:
for (var i = 0; i<= 100; i++){
if( i%3 == 0){
console.log("fizz");
} else {
console.log(i);
}
}
Tiny disclaimer: Up to this point we have broken the problem into parts and I want to demonstrate a regular thought process that we use as developers, I know that we are still missing the condition for 5, and the condition for multiples of both 3 and 5.

Johnathan Wenske
3,410 PointsOkay! Go for it, I want to be a developer, so thinking like one would probably help. I do have a question. The i%3 == 0 is throwing me off. What is a modulo? and What does the % mean?
Sorry for all the questions, I just can't use something unless I know what it does.

Jorge Eduardo Garcia Celorio
10,112 PointsI typed console.log("i"), instead of console.log(i). It is already corrected ;) i is our variable, not the string "i".

Jorge Eduardo Garcia Celorio
10,112 PointsHello Johnathan!
Before I start giving you hints, samples or even complete solutions to your project, tell me.
Into how much depth do you need help?
Do you need a general idea in how to start, or the pseudo code for certain parts of the program, or the step-by-step guide in how to solve it?

Johnathan Wenske
3,410 PointsCould you take me through step by step? It would be so helpful.

Jorge Eduardo Garcia Celorio
10,112 PointsOk, let's break the problem into parts. First, we need to print to the console numbers 1 to 100 using a loop. Can you do it? If you say no, I'm pasting the code to do so. (I just want to measure your programming level, don't worry, I'm helping you out ;) )

Jorge Eduardo Garcia Celorio
10,112 PointsSure, because in the last example you are printing the i value twice (in both the 3/fizz block and the 5/buzz block).
Now, here comes the final tweak.
We already know how to print the fizz numbers separately, as well as the buzz numbers, but when we add the two blocks together we do not get the result that we want. So we need to compare AT THE SAME TIME that i is both a multiple of 3 AND a multiple of 5. If so, we print "fizzbuzz". Then, if i is just a multiple of 3, we print "fizz", and if it is just a multiple of 5 we print "buzz". If all these conditions are not met, we simply print i.
In order to compare two conditions at the same time we use AND which is &&. So if i is a multiple of 3 AND a multiple of 5, we print fizzbuzz. Here's the final code. Let me know if you have further questions:
for (var i = 1; i <= 100; i++){
if (i%5 == 0 && i%3==0){
console.log("fizzbuzz");
} else if (i%3==0){
console.log("fizz");
} else if (i%5==0){
console.log("buzz");
} else {
console.log(i);
}
}

Johnathan Wenske
3,410 PointsIn (i%5 == 0 && i%3==0)
, why don't you use just one &?
Other than that, I understand how to do this now! Thank you so much. I still have so much to learn though.

Jorge Eduardo Garcia Celorio
10,112 PointsOk, well done for your first day!
Deciding which type of loop you need is already an achievement! Congrats!
Now, let's continue.
A for loop uses three conditions for ( "index variable that will count each iteration", "condition under which the loop will perform its inner code", "increase the index variable")
So, to do this you need:
for(var i = 1; i <= 100; i++){
console.log(i);
}
We are initializing with 1 our index "i" (in your case "counter"). Then our condition is that i has to go from 1 all the way to 100, and then we increase i by 1. Inside the brackets we print to the console the current value of the index. SO in the first iteration 1 is printed, and since the variable is increased by 1 and its value will be 2, the loop will run again. ANd so forth until we get until number 100.
Questions? Let me know! :D

Johnathan Wenske
3,410 PointsOkay I think I got it. One question though: Could you please break down the i++ for me? It's throwing me off a little.

Jorge Eduardo Garcia Celorio
10,112 PointsSure!
i++ is the same as typing i = i + 1.
You want to increase the value of i just by 1, so a common practice among developers is to instead of writing i = i + 1, you write i++ (is the same as i = i + 1, but cleaner).
Remember: We want to increase the variable i by 1, so that every time the cycle iterates we print 1, then 2, then 3... until 100.

Johnathan Wenske
3,410 PointsAh! Okay cool, I understand now! Thanks.
So now the rest of the problem.... :|

Jorge Eduardo Garcia Celorio
10,112 PointsDon't worry! Ask me anything you want! I'm here to share with you!
The modulo operator % gets the remainder of a division. For example, if you divide 6 by 3, the answer (quotient) would be 2, and the remainder 0, since 6 is completely divided by 3. Another example would by 24 by 2, where the answer is 12, and the remainder is 0. In the case of 16 divided by 3, the remainder would be 1 since 16 cannot be completely divided by 3. It means that 3 divides 16 up to 5 (part of the answer) and 1 unit remains.
So, we are using i%3 and comparing it to 0 (i%3 == 0) in order to check if the remainder of i divided by 3 is 0. If it is we print "fizz". If it is not, we just print i.
Try doing the same conditional block for i%5 where instead of printing "fizz", you'd need to print "buzz"

Johnathan Wenske
3,410 PointsOkay here is what I did and it didn't work. I figured it was generally the same as using multiples of 3, so I replaced 3 with 5 and fizz with buzz.
for (var i = 0; i<= 100; i++){
if( i%3 == 0){
console.log("fizz");
} else {
console.log("i");
}
if( i%5 == 0){
console.log("buzz");
} else {
console.log("i");
}
}

Jorge Eduardo Garcia Celorio
10,112 PointsAs I previously corrected, don't type console.log("i"), but console.log(i), since you want to print the value of i, not the string "i".
I'm typing here three ways to develop our solution to the problem. The first try is just printing 3/fizz up to 100. The second try is printing 5/buzz up to 100. The final solution is just combining both which as you may see is not what we want, but I will help you tie everything together in my next post.
First try:
for (var i = 1; i <= 100; i++){
if (i%3 == 0){
console.log("fizz");
} else {
console.log(i);
}
}
Then try:
for (var i = 1; i <= 100; i++){
if (i%5 == 0){
console.log("buzz");
} else {
console.log(i);
}
}
Finally try:
for (var i = 1; i <= 100; i++){
if (i%3 == 0){
console.log("fizz");
} else {
console.log(i);
}
if (i%5 == 0){
console.log("buzz");
} else {
console.log(i);
}
}
Can you see what the problem is in the last code example?

Johnathan Wenske
3,410 PointsWhen I view it in the browser it doesn't replace the numbers, it just puts it before or after them. For example: 61 62 fizz 63 64 65 buzz fizz 66 67.....

Jorge Eduardo Garcia Celorio
10,112 PointsBecause the AND operator, by JavaScript standards is always written as &&. The OR operator (which evaluates whether one condition OR the other is true), is ||. NOT EQUAL is !=. As you may see,AND, OR and NOT EQUAL they all come in pairs.
It may be a little dense for you now, but here's the documentation on JavaScript's logical operators.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_Operators

Johnathan Wenske
3,410 PointsAwesome! Thanks again :]

Jorge Eduardo Garcia Celorio
10,112 PointsAnytime Johnathan! If you need further help, don't hesitate to contact me. I'm on twitter at @jegcelorio!
If you thought "how can this ever occur to me" while doing this project, don't worry. It is perfectly normal! More often than not I feel exactly the same way when I see other developers at work. Just keep practicing and developing projects on your own. If you need more practicing/learning resources let me know.
Feel free to ask anything related to both web and game development!
Johnathan Wenske
3,410 PointsJohnathan Wenske
3,410 PointsOkay, Jorge Eduardo Garcia Celorio, I'm a beginner. Just started JavaScript today.......... But I'll try!
for (var counter=100; counter; counter= counter -1)
??????