Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial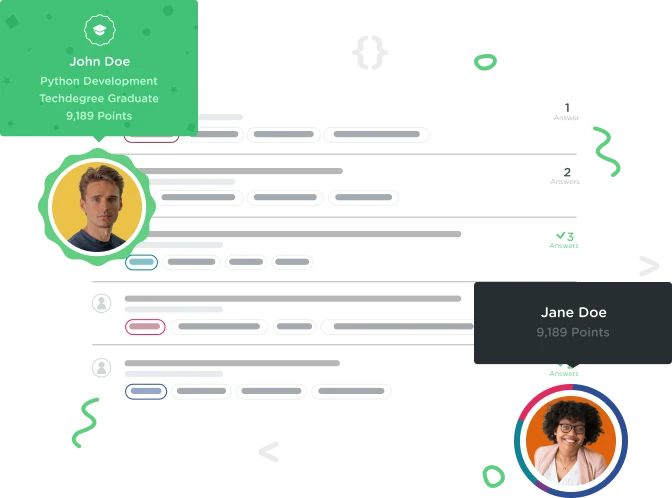
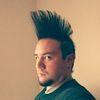
Ben Morse
6,068 PointsProgramming Question: I don't fully understand the "Return" function.
I have been do some java classes here and I thought I knew what the return function was used for, but I feel like I'm just throwing it around in my code just to make sure I don't miss some. Like a "just in case". There are times when i should be using Void, instead of stating a data type and a return.
I'm just looking for someone to make sense of it. When to use a Void and when to use the Return in my code. I don't have an example to post to show what I mean, but if you have some code to shed some light on this then thanks in advance. :)
3 Answers
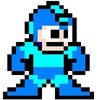
Robert Richey
Courses Plus Student 16,352 PointsHi Ben,
As the author of the program, it's really up to you if a function should return a value or not. Often, though, it is a good idea to return something, so that you can use that value somewhere else in your code.
Functions that don't return a value (also known as Procedures) have limited use, because you can't really do anything with it other than call it and let it do whatever it does.
int foo = 0;
public void add_procedure(int a, int b) {
foo = a + b;
}
public int add_function(int a, int b) {
return a + b;
}
add_procedure(3, 5);
// ok, now what? unless you know about foo and how to access it, it is gone
int result = add_function(3, 5);
// it is immediately clear that result is holding the value returned by add_function(),
// now you can do whatever you want with that value.
// you can use the function in any expression that can take an int
int x = 5 + add_function(3, 5);
System.console.log("The result from adding 3 and 5 is: " + add_function(3, 5));
In summary, functions that return a value are dramatically more useful than those that don't. Unless you have a really good reason to not return a value, try to always do so - even if it's just a boolean to convey whether the function call succeeded or not.
Best Regards
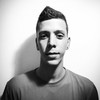
Jesus Mendoza
23,289 PointsIf a function does not return a value, the return statement is used to break the function (to exit the function when it finish), if the function returns a value, the return statement is used to return the value of that function.
So, for example, if you create a function that checks if you connected to a data base successfully, you can return true or false. If you made a function to do some math, you can use return value

Dan Johnson
40,533 PointsA rule of thumb you can follow on whether or not a method should return a value is if it modifies the state (member variables / properties) of the object. If it does, it probably shouldn't return any value (void). If not, then it's probably requesting information about the state of the object (return something).
Of course there are always exceptions.
Further reading: http://martinfowler.com/bliki/CommandQuerySeparation.html