Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial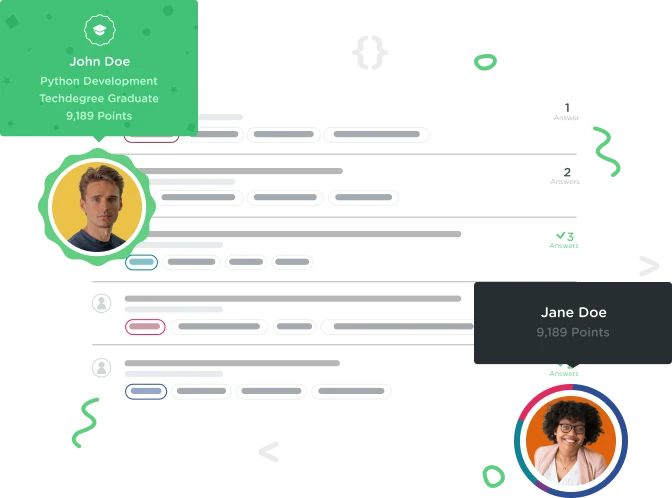
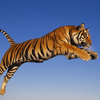
Diego Murray
2,515 PointsProject Euler Problem 10 JAVA - Bug
Not sure why I don't get the right answer here...
public class Main {
public static final int num = 2000000;
public static void main(String[] args) {
int sum = 0;
for (int i = 0; i < num; i++) {
if(isPrime(i)) {
sum += i;
}
}
System.out.println("Sum: " + sum);
}
public static boolean isPrime(int n) {
if (n <= 1) {
return false;
}
for (int i = 2; i < Math.sqrt(n); i++) {
if (n % i == 0) {
return false;
}
}
return true;
}
}
1 Answer

Simon Coates
28,694 PointsWorking on sums of primes under 10. Starting small so numbers are manageable.
public class Main {
public static final int num = 10;
public static void main(String[] args) {
int sum = 0;
for (int i = 0; i < num; i++) {
if(isPrime(i)) {
sum += i;
System.out.println("Prime:"+i);
}
}
System.out.println("Sum: " + sum);
}
public static boolean isPrime(int n) {
if (n <= 1) {
return false;
}
for (int i = 2; i < Math.sqrt(n); i++) {
if (n % i == 0) {
return false;
}
}
return true;
}
}
produces
Prime:2
Prime:3
Prime:4
Prime:5
Prime:7
Prime:9
Sum: 30
I'm guessing
public class Main {
public static final int num = 10;
public static void main(String[] args) {
int sum = 0;
for (int i = 0; i < num; i++) {
if(isPrime(i)) {
sum += i;
System.out.println("Prime:"+i);
}
}
System.out.println("Sum: " + sum);
}
public static boolean isPrime(int n) {
if (n <= 1) {
return false;
}
for (int i = 2; i <= Math.sqrt(n); i++) {
if (n % i == 0) {
return false;
}
}
return true;
}
}
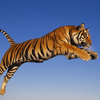
Diego Murray
2,515 PointsI see now that it's collecting numbers that aren't Primes( ex. 2, 4). You posted the same code again, not sure if that was intentional..

Simon Coates
28,694 Pointsthere was a difference in the isPrime method. <= not <
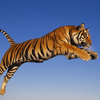
Diego Murray
2,515 PointsI see now. Thank you
Diego Murray
2,515 PointsDiego Murray
2,515 PointsLooking for sum of all primes under 2 million.