Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial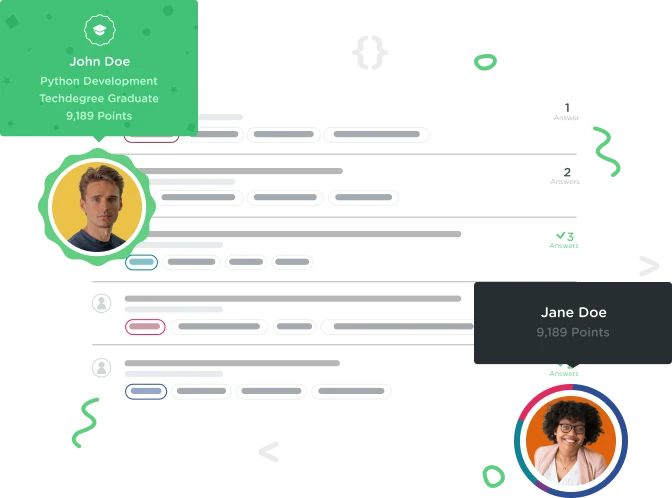
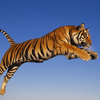
Diego Murray
2,515 PointsProject Euler Problem 4
A palindromic number reads the same both ways. The largest palindrome made from the product of two 2-digit numbers is 9009 = 91 × 99.
Find the largest palindrome made from the product of two 3-digit numbers.
Can someone please help me debug this? It is resulting in 580085.
public class Problem4 {
public static int createPalindrome() {
for(int i = 999; i > 100; i--) {
for(int j = 999; j > 100; j--) {
int prod = i*j;
String s = Integer.toString(prod);
String s2 = new StringBuffer(s).reverse().toString();
if(s.equals(s2)) {
return prod;
}
}
}
return 0;
}
public static void main(String[] args){
int answer = createPalindrome();
System.out.println(answer);
}
}
4 Answers
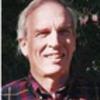
jcorum
71,830 PointsDiego, what the added part is doing is keeping track of the largest palindrome. Each time the inner loop finds a palindrome it then compares it to the previous largest one (which is stored in max), and if the new palindrome is larger then it puts it in max, replacing the former largest one. So at the end of the loops max will have the largest palindrome.
Hope this helps!
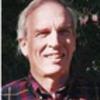
jcorum
71,830 PointsDiego, sorry about that. Misread your question. Here, try this:
public static int createPalindrome() {
int max = 0;
for(int i = 999; i > 99; i--) {
for(int j = 999; j > 99; j--) {
int prod = i*j;
String s = Integer.toString(prod);
String s2 = new StringBuffer(s).reverse().toString();
if(s.equals(s2)) {
if (prod > max) { //I added this part
max = prod;
}
}
}
}
return max;
}
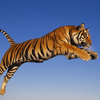
Diego Murray
2,515 PointsWow, thank you for your quick responses. Can you please briefly explain why this added part is necessary? Thanks!

Anjali Pasupathy
28,883 PointsThe added part is necessary because prod doesn't just decrease with each iteration of the for loops. It starts at 999*999, and then steadily decreases to 999*101=110889. After that, j jumps back up to 999 and i decreases by 1, so the next value prod takes is 998*999 = 997002. Basically, your code starts prod at its highest possible value, steadily decreases that value, and then suddenly elevates the value up to the next highest value prod can take. Because of this, you need to keep track of the max palindromic product using the max variable, before returning max at the end.
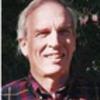
jcorum
71,830 PointsDiego, you just need to change the for loop start and end points so they are for 2-digit numbers rather than 3-digit numbers:
public static int createPalindrome() {
for(int i = 99; i > 9; i--) {
for(int j = 99; j > 9; j--) {
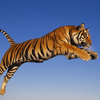
Diego Murray
2,515 PointsThe problem says, "Find the largest palindrome made from the product of two 3-digit numbers." The two 2-digit numbers served as an example.

Anjali Pasupathy
28,883 PointsI think what's going wrong is the structure of your for loops. Your for loops start with i at 999, and iterate j from 999 to 101 before decrementing i. The last value prod takes before decrementing i is 999*101 = 110899. The first value prod takes after decrementing i is 998*999 = 997002, which is larger than 999*101. Basically, your code starts prod at its highest possible value, steadily decreases that value, and then suddenly elevates the value up to the next highest value prod can take. I would recommend making the following adjustments to account for that: rather than returning prod, store prod in some variable that keeps track of the palindromic products (I'm going to call this value palinProd). Then, inside the innermost loop, compare that palinProd to prod. If prod becomes less than or equal to palinProd, break the innermost loop. If, before that happens, prod turns out to be a palindrome, set palinProd to prod.
public class Problem4 {
public static int createPalindrome() {
int palinProd = 0;
for(int i = 999; i > 100; i--) {
for(int j = 999; j > 100; j--) {
int prod = i*j;
if (prod <= palinProd) {
break;
}
String s = Integer.toString(prod);
String s2 = new StringBuffer(s).reverse().toString();
if(s.equals(s2)) {
palinProd = prod;
}
}
}
return palinProd;
}
public static void main(String[] args){
int answer = createPalindrome();
System.out.println(answer);
}
}
I can't check this code in java at this moment, so I can't guarantee that it'll work. But I'm fairly certain the algorithm I laid out should get you the answer you're looking for.
I hope this helps!