Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial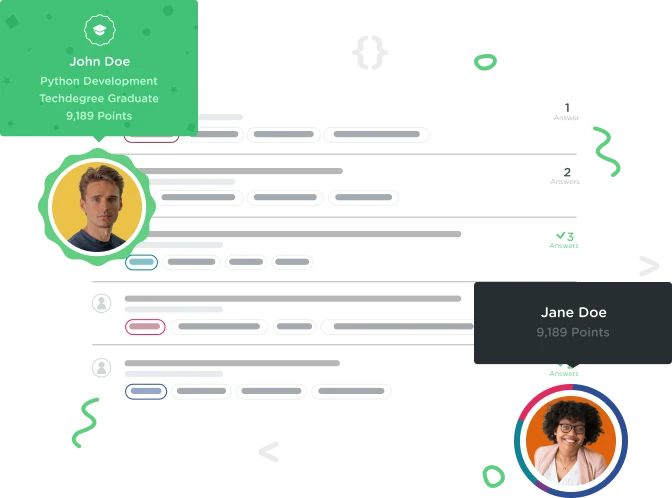
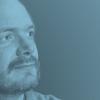
Edmund Southgate
12,056 PointsPromises and calling asynchronous console applications in Node.js
Hello, I have an asynchronous function that goes out to an API and gets the current exchange rate. It passes the unit test proving that it returns a number. However when I try to run it in node in the terminal my code doesn't work. I think because it is asynchronous. I have tried writing it as a promise but that doesn't seem to work.
So the app.js file looks like this...
const getExchangeRate = require('./tip_calculator/tip_calculator_methods.js').getExchangeRate;
let currency1 = process.argv.slice(2, 3)[0];
let currency2 = process.argv.slice(3, 4);
const logExchangeRate = new Promise(function(resolve, reject) {
getExchangeRate(currency1, currency2).then(function(data) {
resolve(data);
}).catch(console.log('Oops there has been an error!'))
});
logExchangeRate.then(function(value) {
console.log('The exchange rate is ' + value);
}).catch(console.log('There has been an error'));
The exchange rate function itself looks like this...
function getExchangeRate(currencyFrom, currencyTo) {
//connect to API url
let exchangeRatePromise = new Promise((resolve, reject) => {
const exchangeRateRequest = http.get(`http://api.fixer.io/latest?base=${currencyFrom}`, response => {
let body = "";
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
const exchangeRates = JSON.parse(body);
if(exchangeRates.error) {
reject(new Error('Request to server failed'));
} else {
const exchangeRate = exchangeRates.rates[currencyTo];
resolve(exchangeRate);
}
});
exchangeRatePromise.then((data) => {
return data;
}).catch((error) => {
return error;
})
});
});
}
I've tried everything I can think off and probably made a terrible mess of the code in the process. It seems odd to be calling a function that is already a promise inside another promise. However the then method doesn't work if I simply try to call the method in the app js file. I hope this made some sense to someone and that you can prevent me throwing my MacBook out of the window.
Edmund Southgate
12,056 PointsEdmund Southgate
12,056 PointsIt used to work when I was using callback functions instead of promises...but the full application will get the exchange rate then convert the bill so it makes sense to use promises when I write that code. Perhaps I should just trust my unit test instead of manually testing it in command line?