Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial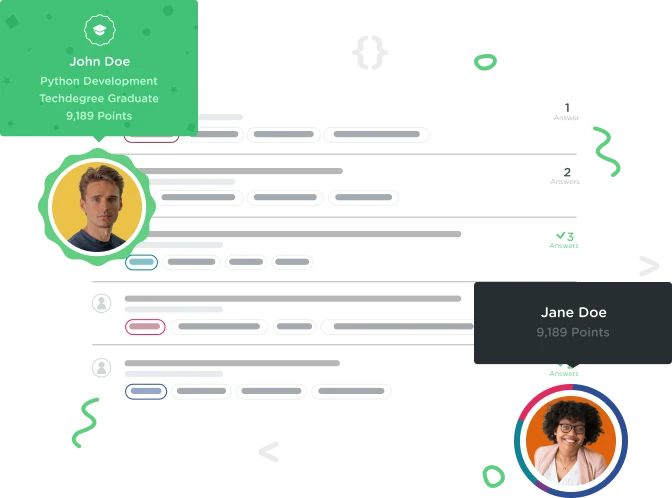

ju5t
961 PointsPromises and multiple calls
I'm working on a project that connects to a message queue. Once it receives a message it has to run the following steps in sequence (for a single task):
- Connect to a database and retrieve the row for its task;
- Download a file from any storage (local or s3);
- Extract the file into a temporary location;
- Run analysis on the contents of that file;
- Generate images and store them (local or s3);
- Update the database.
This turned out to be a major challenge for a Javascript newbie. All those asynchronous calls are mind blowing. I have no problem with all the individual tasks but I can't get them to run in the right sequence. I'm struggling how to fit this into Promises.
I've done the course on both the basics of NodeJS and the one that explains Promises but what I find most difficult is the fact that each step in this sequence has it's own asynchronous call. I find that I'm quickly building my own version of a pyramid of doom.
I can't share all code but I've added a gist on GitHub: https://gist.github.com/ju5t/2ee007af55c3dc8b235f3839ec4c2dac
-
database.query
returns a Promise. -
files.get
returns afs.createReadStream
I expected to see:
Extraction done
Inspection {
id: '2ce8a281-3354-4f67-b41a-b0d8d2466c6b'
etc.
Instead I get:
$ node index.js
undefined
Extraction done
Who can guide this poor Python-developer in the right direction?
1 Answer

ju5t
961 PointsI think I found the solution myself. In the example I rewrote
.then(inspection => {
files.get(`${inspection.id}.zip`)
.pipe(unzip.Extract({path: `tmp/${inspection.id}/`}))
.on('close', () => {
console.log('Extraction done');
return inspection;
});
})
into
.then(inspection => {
return new Promise((resolve, reject) => {
var file = files.get(`${inspection.id}.zip`);
file.pipe(unzip.Extract({path: `tmp/${inspection.id}/`}))
file.on('close', () => {
console.log('Extraction done');
resolve(inspection)
})
});
})