Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial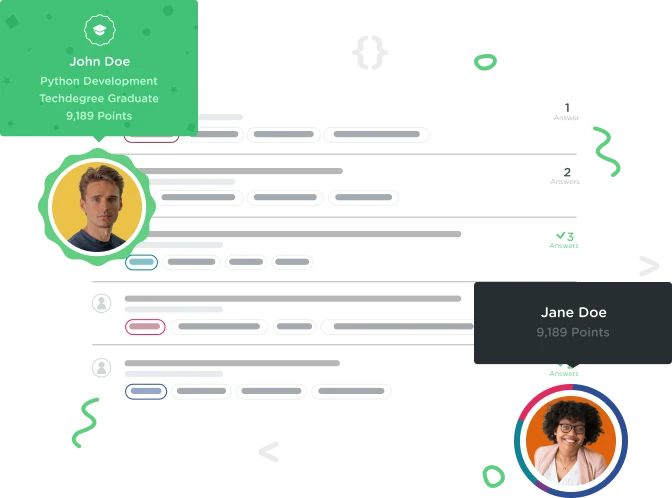

Laurence kite
11,768 PointsPromises and Video by Chalkey
I am confused by this video mainly in terms of how Promises work and how it all fits together:
Here is some code its not marked up properly but hope it is ok:
function getJSON(url) { return new Promise(function(resolve, reject) { var xhr = new XMLHttpRequest(); xhr.open('GET',url); xhr.onreadystatechange = handleResponse; xhr.onerror = function(error) { reject(error);} xhr.send();
function handleResponse() {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
var employees = JSON.parse(xhr.responseText);
resolve(employees)
}else {
reject(this.statusText);
}
}
}
}); }
var ajaxPromise = getJSON("../data/employees.json");
function generatListItems(employees) { var statusHTML = ''; for (var i=0; i<employees.length; i += 1) { if (employees[i].inoffice === true) { statusHTML += '<li class="in">'; } else { statusHTML += '<li class="out">'; } statusHTML += employees[i].name; statusHTML += '</li>'; }
return statusHTML;
}
function generateUnorderedList(listItems) { return '<ul class="bulleted">' + listItems + '</ul>'; }
function addEmployeesToPage(unorderList) { document.getElementById('employeeList').innerHTML = unorderList; }
ajaxPromise.then(generatListItems) .then(generateUnorderedList) .then(addEmployeesToPage) .catch(function(e) { console.log(e); });
xhr.onreadystatechange = handleResponse;
This line is a bit confusing since the function called to handle the readystatechange is assigned to the handleResponse function.. but functions are normally called by handleResponse(); with the brackets.. but is it because the event only calls the function so it is not called here.. just named as a handler
Also can someone explain why this code seems so much harder to read then the previous code..
Why is the Promise returned ..