Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial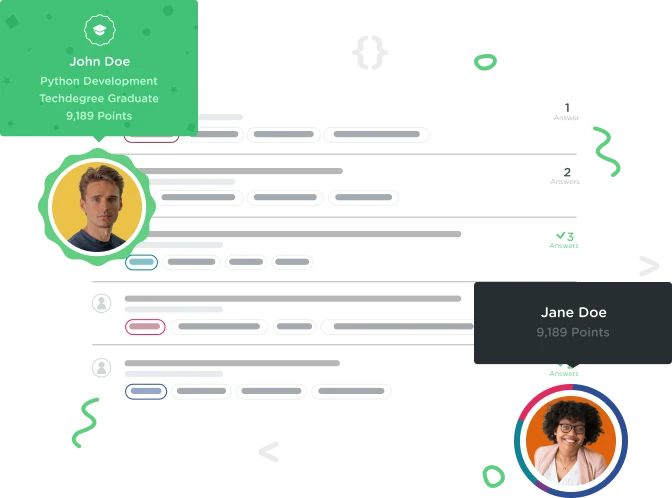
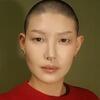
Begana Choi
Courses Plus Student 13,126 Pointspromises.js:19 XHR failed loading: GET "https://en.wikipedia.org/api/rest_v1/page/summary/Anatoly%20Ivanishin".
I have some error issues that always with wiki url. and before I use Promise.all() everything worked but not perfectly, for example, one of the Promise got error for the same reason. and now I can't use Promise.all() at all. (also I checked previous replies and nothing worked for me) what can I do?
3 Answers
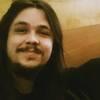
Nikola Ivanovic
8,786 PointsHi! I had the same problem I resolved it by following this discussion here. In function getProfiles you need to insert conditional statement like this:
if (person.name == "Anatoly Ivanishin") {
person.name = "Anatoli_Ivanishin"
}
So the final example of the getProfiles function would be :
function getProfiles(json) {
const profiles = json.people.map(person => {
if (person.name == "Anatoly Ivanishin") {
person.name = "Anatoli_Ivanishin"
}
return getJSON(wikiUrl + person.name);
});
return Promise.all(profiles);
}
The name in the JSON file on the notify.org was 'Anatoly' instead 'Anatoli' like the name on Wikipedia. Hope this helps.

Martin Coton
3,015 PointsI'm looking for the rsolution to this that doesn't require a hard-coded conditional statement. This method is a hack not a fix. Has anyone found one, I've tried this (which doesn't work):
function getProfiles(json) {
const profiles = json.people.map( person => {
const profile = getJSON(wikiUrl + person.name)
.then(profile => profile)
.catch(e => e);
});
return Promise.all(profiles)
.then(profiles)
.catch( function(err) {
console.log('Promise was rejected', err);
return(profiles);
});
}
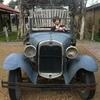
Brendan Moran
14,052 PointsUse Promise.allSettled().
function getWikiProfiles(data) {
const profiles = data.people.map(person => {
return getJSON(wikiUrl + person.name);
});
return Promise.allSettled(profiles);
}
function generateHTML(data) {
//console.log(data);
data.forEach(person => {
if (person.status === 'fulfilled') {
person = person.value;
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
}
});

Sean Gibson
38,363 PointsThank you, Nikola Ivanovic! In case anyone is having this problem again: recently Yulia Peresild joined the list of people in space, but the open-notify api data was misspelled as "Yulia Pereslid", which was causing me problems. Nikola's solution worked for me. Some people are claiming it's a hack, which may be true, but I'm happy with that, as it allows me to continue to code along with the teacher.
Begana Choi
Courses Plus Student 13,126 PointsBegana Choi
Courses Plus Student 13,126 PointsThank you so much it works perfectly!!
Zachary Danz
Front End Web Development Techdegree Graduate 15,024 PointsZachary Danz
Front End Web Development Techdegree Graduate 15,024 PointsThank you for this comment, extremely helpful!
Hamdi Elshahat
2,565 PointsHamdi Elshahat
2,565 PointsI was going crazy, you saved me!