Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial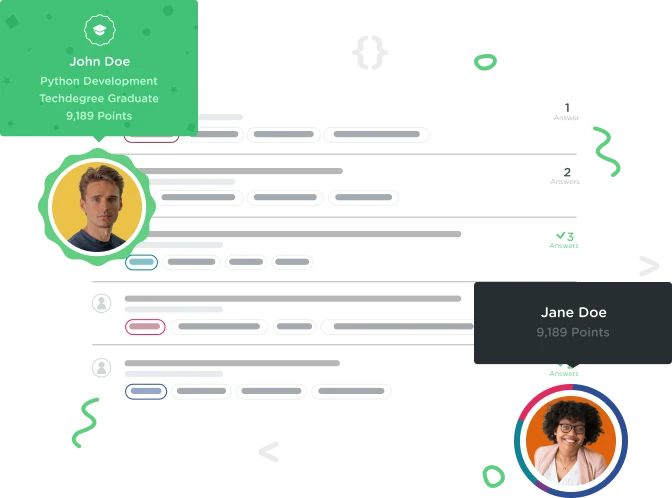

mustafa dogan
4,391 PointsPrompt and string relation is incorrect!
Prompt and string relation is correct only for addition, when you do subtraction it works- mean prompt returns number when use them for othe operation other than addition.
Here is the example:
var theDateYouBorn= prompt(" when did you born");
var year=prompt(" which year is now");
var age= year-theDateYouBorn;
document.write("Your are "+age+" old");
2 Answers

LaVaughn Haynes
12,397 PointsThe prompt returns strings that look like numbers but are still strings. When you use subtraction, javaScript coerces or forces the strings into numbers. When you use addition, javaScript concatenates the values because
string1 + string2 = string1string2
The solution is to use parseInt() to convert those strings into numbers. If you do that then addition will work as well.
var theDateYouBorn = prompt(" when did you born");
//outputs "string"
document.write('<p>' + typeof theDateYouBorn + '</p>');
//convert string to number
theDateYouBorn = parseInt(theDateYouBorn, 10);
//outputs "number"
document.write('<p>' + typeof theDateYouBorn + '</p>');
var year = prompt(" which year is now");
//again, convert string to number
year = parseInt(year, 10);
var age = year - theDateYouBorn;
document.write("Your are " + age + " old");
Check here for more information on parseInt https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt

mustafa dogan
4,391 PointsThank you for reply. The sentence of "Js forces string to number" makes sense to me:)

LaVaughn Haynes
12,397 PointsSorry :)
Yes. You want to force JS to read the variable as a number.
If you do this:
var myNumber = prompt("Enter a number");
myNumber will be a string. Sometimes javaScript will treat it like a string.
"+" can be used with numbers and strings.
String
myNumber + " James";
Below it makes no sense as a string so javaScript will guess that you want a number and force it to a number
Number
myNumber - 8;
You can make sure that javaScript doesn't have to guess by specifically telling javaScript that it is a number like this:
myNumber = parseInt(myNumber, 10);
Now javaScript won't guess or force it into something else and you wont get unexpected results