Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial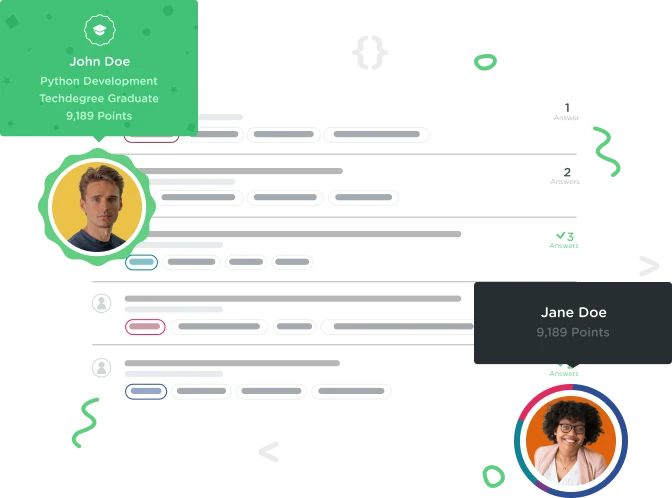
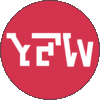
syalih
16,468 PointsPrompter.java
I seriously dont understand what is going on in this prompter here is the code if any one could explain it would be help full for me please here is the code
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play() {
while (mGame.getRemainingTries() > 0) {
displayProgress();
promptForGuess();
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n",
mGame.getRemainingTries(),
mGame.getCurrentProgress());
}
}
2 Answers

Nikolay Mihaylov
2,810 PointsThe problem is that any outcome of the promptForGuess() method, short of an exception being thrown, will result in a true value for isValidGuess. So after the jvm runs the method once the value will be true no matter if the user has guessed correct or not. This is because the result of applyGuess() goes into isHit. isHit is then returned by promptForQuess() inside the paly() method. Then play() doesn't use this value at all.
So what happens is that program will ask the user for a guess once. No matter if the quess is true or not it will flag it as true. Then as far as I can see it will enter an infinite loop since the tries can't go down to zero (assuming they are reduced each time appyGuess() is called).
This is my revised version:
import java.io.Console;
public class Prompter {
private Game mGame;
private Console console;
private boolean isValidGuess = false;
public Prompter(Game game) {
mGame = game;
console = System.console();
}
public void play() {
while (mGame.getRemainingTries() > 0 && !isValidGuess) {
displayProgress();
promptForGuess();
}
}
public void promptForGuess() {
String guessAsString = console.readLine("Enter a letter: "); // If not ask for a quess
try {
isValidGuess = mGame.applyGuess(guessAsString);
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage()); // Throw exception if console input is bad.
}
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n", mGame.getRemainingTries(), mGame.getCurrentProgress());
}
}
I have totally removed isHit. Instead the applyGuess() return is stored in isValidGuess. isValidGuess is now declared as global variable for the object. promptForGuess() no longer returns a value The play method checks both the remaning tries and the value of isValidGuess
Console is also defined as a global variable and only initialized once in the constructor.
If you have any questions feel free to ask!
P.S.
Next time you post comment your code as well code! :)
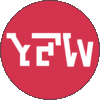
syalih
16,468 PointsThank You :) but what is this I dont understand private Game mGame; private Console console; private boolean isValidGuess = false;
public Prompter(Game game) { mGame = game; console = System.console(); }

Nikolay Mihaylov
2,810 Pointsprivate Game mGame;
Declares a variable with the type Game.
private Console console;
Declares a variable with the type Console. In a nutshell the console object allows the java program acccess to the underlying platform console. So for example if you are running this program from Windows Command Prompt, the console object allows your JVM and thus your program to output to the platform console ( ie. Command Prompt ).
private boolean isValidGuess = false;
Declares a boolean ( true/false ) variable and initializes ( sets ) its value to false;
public Prompter(Game game) {
mGame = game;
console = System.console();
}
This is a java constructor for the Prompter object. So apart from all the underlying shenanigans that occur when creating an object ( such as allocating physical memory) the program also calls the constructor. Inside of it, a programmer can give a set of instructions, to be executed, when the object is created. So what I did here is initialized the value of mGame with the constructor argument and the console object using the System.console() method.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherAre you looking for a line by line breakdown?