Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial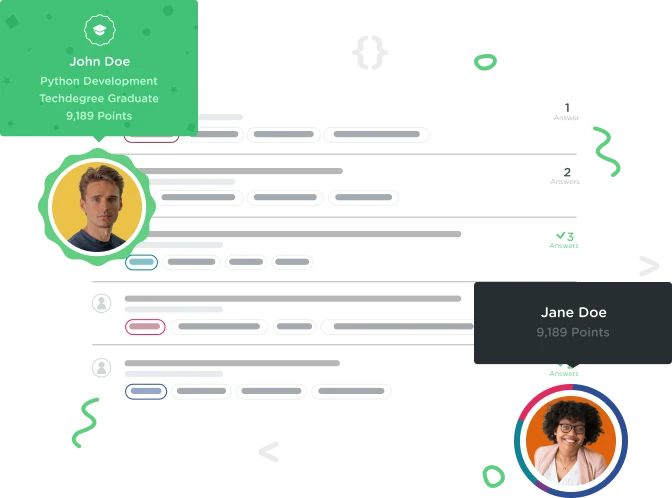

ISAIAH S
1,409 Points./Prompter.java:24: error: cannot find symbol isHit = mGame.applyGuess...
Error:
./Prompter.java:24: error: cannot find symbol
isHit = mGame.applyGuess(guessAsString);
^
symbol: variable guessAsString
location: class Prompter
1 error
Code:
Hangman.java:
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
prompter.play();
}
}
Game.java:
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
private char validateGuess(char letter) {
if (! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed");
}
return letter;
}
public boolean applyGuess(String letters) {
if (letters.length() == 0) {
throw new IllegalArgumentException("No letter found.");
}
return applyGuess(letters.charAt(0));
}
public boolean applyGuess(char letter) {
letter = validateGuess(letter);
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
}
Prompter.java:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play() {
while(mGame.getRemainingTries() > 0) {
displayProgress();
promptForGuess();
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
}
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.", iae.getMessage());
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n",
mGame.getRemainingTries(),
mGame.getCurrentProgress());
}
}
2 Answers
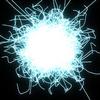
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsI spent about 20 minutes going through your game class and comparing it to mine, even coping yours in and compiling it and the class complied, so that is very odd. However I have copied my game class below, try to insert this and see if it works, very odd.
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public String getAnswer() {
return mAnswer;
}
private char validateGuess(char letter) {
if(! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + "has already been guessed");
}
return letter;
}
public boolean applyGuess (String letters) {
if (letters.length() == 0) {
throw new IllegalArgumentException("No letter found");
}
return applyGuess(letters.charAt(0));
}
public boolean applyGuess(char letter) {
letter = validateGuess(letter);
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter : mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
}
Let me know if this clears up the error, but I couldn't find it in your code.
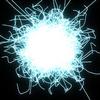
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsOkay, I looked over your code with a fine tooth comb, it looks like you had it right the first time with your line of
isHit = mGame.applyGuess(guessAsString);
Sorry I didn't see that you had correctly declared the variable above it. Looking over it I see there is an closing curly brace above your first try block as GuessAsString,
I think this might also be the problem as well.
You want to keep your while block open during this until it is still a valid guess, sorry to run you in circles on this I gave it a better look,
Let's try this.
public static boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess =true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
Use that as your for promptForGuess, it is much like your's except it is removing the extra curly brace.
Let me know if this helps, if not I'll be back in touch till we get this worked out.

ISAIAH S
1,409 PointsI changed
try { isHit = mGame.applyGuess(guessAsString); isValidGuess = true;
to
try { isHit = mGame.applyGuess(String guessAsString); isValidGuess = true;
but I am getting the following errors:
./Prompter.java:24: error: ')' expected
isHit = mGame.applyGuess(String guessAsString);
^
./Prompter.java:24: error: illegal start of expression
isHit = mGame.applyGuess(String guessAsString);
^
./Prompter.java:24: error: cannot find symbol
isHit = mGame.applyGuess(String guessAsString);
^
symbol: variable String
location: class Prompter
3 errors
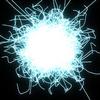
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello I apologize, I could have sworn I responded but it appears that I have not, were you able to get this sorted out in work spaces?

ISAIAH S
1,409 PointsNo.
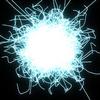
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Isiah, I gave your code a second look and updated my answer, let me know if this sorts it out.

ISAIAH S
1,409 PointsI changed
public static boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
to this
public static boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
try {
isHit = mGame.applyGuess(guessAsString);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
but I am getting the following error:
Prompter.java:24: error: non-static variable mGame cannot be referenced from
a static context
isHit = mGame.applyGuess(guessAsString);
^
1 error
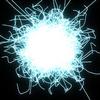
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello, that is actually good news. We got past one error and onto the next.
This one is a an easy fix, in your Prompter Java class towards the top where you are defining the mGame Variable, it is the towards the very top, it looks like.
private Game mGame;
Give it the keyword "static" which allows it to be referenced inside your loops
So it should look something like
private static Game mGame;

ISAIAH S
1,409 PointsNow it says it's missing a return statement.
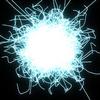
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello, this is still good news. It means we're working through the errors and getting it to work, what error does it toss?
Can you copy it?

ISAIAH S
1,409 Points./Game.java:58: error: missing return statement
}
^
1 error
ISAIAH S
1,409 PointsISAIAH S
1,409 PointsIt worked!!! :-)
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsGood news!
Happy to hear it, it must have been something so minor, or just a glitch in workspaces, because when I tried what you initally copied over to me it compiled since all your edits were to the prompter class, at least it worked out!
Let me know if anything else comes up.
mannyaharon
873 Pointsmannyaharon
873 PointsHi rob! I seem to be having the same issue and when I compile I get the same error. What's weird is that I can still run the program but all that will happen is I will be prompted for a letter and nothing will happen, it just loops indefinitely until I hit control 'c'. I even copied your code after going through my code for an hour because I wanted to see what would happen, and I still get the same error. Not sure what to do from here :( -emanuel
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Emanuel can you copy your code? I'll give it a look.
mannyaharon
873 Pointsmannyaharon
873 PointsThanks for your Rob, Here is my code...
Hangman Class:
Game class:
Prompter class:
The error I receive still is:
Thank you for all your help in advance!
Warm Regards, Emanuel