Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial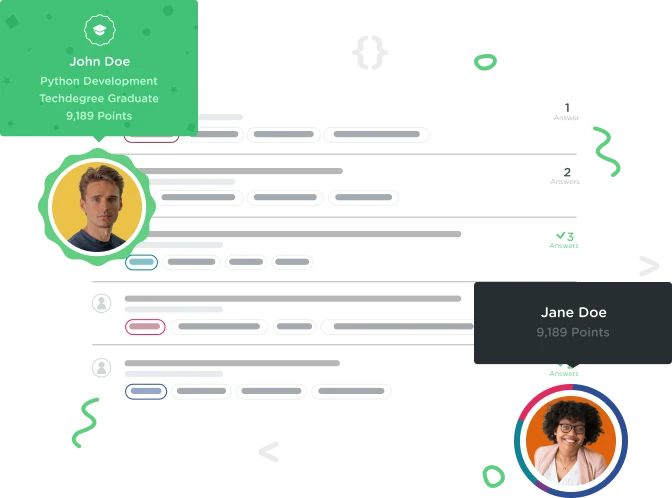
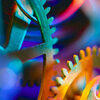
ja5on
10,338 Pointsprop in person
In the video (Use for in
to Loop Through an Object's Properties), this code
for ( let prop in person ) {
console.log(${prop}: ${person[prop]}
);
}
How do I know what prop does? I cant see a link to the object? prop could be any name like a variable the teacher says. It accesses the objects properties but how?
The code says it gains access to the person object but how does it select the properties?
2 Answers
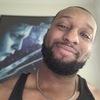
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHi jason7,
prop is simply the variable name that you’re giving to hold each item during each iteration. So if you had an object named myCar, it might have several properties (i.e. “prop”).
let myCar = {
make: “Chevrolet”,
model: “Cruze”,
year: 2019,
color: “Red”,
previousOwners: null
}
// the properties are make, model, etc... | the values are “Chevrolet”, “Cruze”, etc...
for (let prop in myCar) {
console.log(`${prop}: ${myCar[prop]}`); // in order to use template literals you need to surround the string in back tics
}
/* Results
make: Chevrolet
model: Cruze
year: 2019
color: Red
previousOwners: null
*/
/* calling the prop variable targets the object’s properties, the use of bracket notation is a way to target the values that belong to those properties. */
If this still doesn’t make sense let me know and I’ll try again.

Alex Miller
624 PointsThe way i understood 'for ... in' loop is
for (let abc in myCar)
where let abc is a declared variable that holds inside all properties of the object is assigned to, in this case myCar. so if console.log(abc) it will return make, model, year, color, previousOwners as the loop iterates over each individual property of the myCar object.
and if console.log(${abc} : ${myCar[abc]}
); it will return all key:value pairs of the object myCar that the abc variable is linked to, takes a bit to get used to this concept though
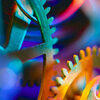
ja5on
10,338 PointsThanks Alex Miller your help is reinforcing my understanding.
ja5on
10,338 Pointsja5on
10,338 PointsBrilliant, like the car format easier to understand. When I run the code:- for (let prop in myCar) { console.log(
${prop}: ${myCar[prop]}
); // in order to use template literals you need to surround the string in back tics } I get a undefined below the print results, any idea why?Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsAre you saying that you get the printed results, but you also get an undefined after the printed results?
ja5on
10,338 Pointsja5on
10,338 PointsOh yes I get the printed results and the undefined but just to clarify all the results print like they are supposed to. Its not a big problem it may be just how the console does things I don't know, I just wondered.
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsNo problem, I just wanted to verify that I knew what was happening.
If you're running the code from above in your browser console, the reason undefined is printed at the end is because the console.log function doesn't return a value.
The same thing would happen if you were to enter the following in your console:
The above statement assigns the string "cheeseburger" to the lunch variable, but it doesn't return a value so the browser has nothing to print. So printing
undefined
is its way (in this particular case) of letting you know that no value is returned.