Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial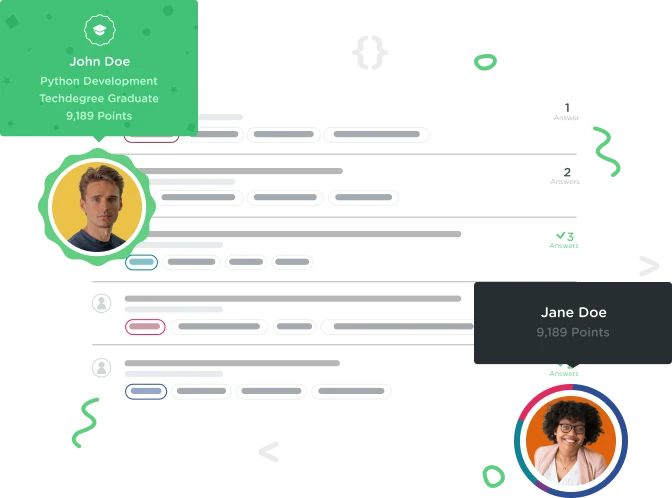
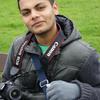
Akib Quraishi
2,306 PointsProperty Based Animation
Instead of fading in the text of the predictionLabel, animate the text down to the bottom of the screen when you a tap or motion event begins. Once the tap or motion event ends then animate the text upward from the bottom of the screen. Hint: Use the animateWithDuration:animations:completion method. You will need to add code to the completion block to wait till the animate finishes.
( From Apple Doc)For example, if you want to fade a view until it is totally transparent and then remove it from your view hierarchy, you could use code similar to the following: [UIView animateWithDuration:0.2 animations:^{view.alpha = 0.0;} completion:^(BOOL finished){ [view removeFromSuperview]; }];
my question are:: What code i need to animate the text down to the bottom of the screen ? what code i need to animate the text upward from the bottom of the screen ?
i need transition for sure.
3 Answers

Stone Preston
42,016 Pointsif you wanted to animate a label to say the bottom of the screen you could call the following method:
[UIView animateWithDuration:1 animations:^{
self.label.frame = CGRectMake(self.label.frame.origin.x, self.view.frame.size.height - self.label.frame.size.height, self.label.frame.size.width, self.label.frame.size.height);}
completion:^(BOOL finished){
//animation complete
}];
which would animate the label to the bottom of the screen over 1 second.
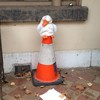
bronnyreinhardt
1,007 PointsTo make it bob down a lil bit and then animate back up, as per the Extra Credit exercise, I did the following:
#pragma mark - prediction
- (void) makePrediction {
[self.backgroundImageView startAnimating];
self.predictionLabel.text = [self.thegrandpa randomPrediction];
AudioServicesPlaySystemSound(soundEffect);
[UIView animateWithDuration:1 animations:^{
self.predictionLabel.frame = CGRectMake(65, 370, self.predictionLabel.frame.size.width, self.predictionLabel.frame.size.height);}
completion:^(BOOL finished) {
[UIView animateWithDuration:1 animations:^{
self.predictionLabel.frame = CGRectMake(65, 347, self.predictionLabel.frame.size.width, self.predictionLabel.frame.size.height);}];
}];
}
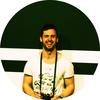
Malte Niepel
19,212 PointsI know it's an older thread, but I hope you guys can still help me. I used your Code Stone and my label disappears. Now since I am new to programming, I can't figure this out after trying. Is it because I can't move the label I added in the storyboard and therefore need to programmatically add a label?
Rashii Henry
16,433 PointsRashii Henry
16,433 Pointshey stone, couldn't you also animate the frame of the label to a CGPoint on the screen? and use CGPointMake(CGRectGetMidX(self.frame) , CGRectGetMidY(self.frame) - (however many pixels far you want to move it down, change to a plus if you want to move it up);
I'm just asking wouldn't it work about the same?
Stone Preston
42,016 PointsStone Preston
42,016 Pointsyou can animate it to any point you want to.