Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial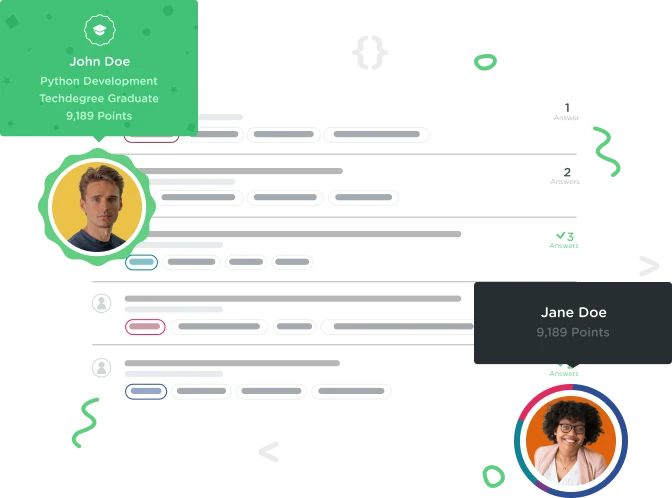
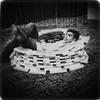
Saad Khan Malik
25,199 PointsProperty error on "https.STATUS_CODES"
app.js
//Problem: We need a simple way to look at a user's badge count and JavaScript points
//Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
var https = require("https");
var username = "saadkhanmalik123";
//print out message
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in JavaScript";
console.log(message);
}
//print out error messages
function printError(error){
console.error(error.message);
}
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response){
var body = "";
//Read the data
response.on('data', function (chunk){
//console.log('BODY: ' + chunk); // get whole body
body += chunk;
});
response.on('end',function(){
if (response.statusCode === 200){
try {
var profile = JSON.parse(body);
/*console.dir(profile); //getting all the profile information*/
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch(error) {
//Pasrse Error Here
printError(error);
}
} else {
printError({message: "There was an error getting the profile for " + username + ". (" + https.STATUS_CODES[response.statusCode] + ")"});
}
});
//Parse the data
//Print the data
});
request.on("error", printError);
Console
treehouse:~/workspace$ node app.js
/home/treehouse/workspace/app.js:38
or getting the profile for " + username + ". (" + https.STATUS_CODES[response.
^
TypeError: Cannot read property '404' of undefined
at IncomingMessage.<anonymous> (/home/treehouse/workspace/app.js:38:113)
at IncomingMessage.emit (events.js:129:20)
at _stream_readable.js:908:16
at process._tickCallback (node.js:355:11)
The carrot point on the opening square bracket.
Thanks in advance!
3 Answers

Seth Kroger
56,413 PointsSTATUS_CODES is one of the few things node's https doesn't replicate from http, and using https in it's place here is causing the error. You'll need to require http as well as https for that one bit.

Sebastian Röder
13,878 PointsThere is an easier way to get the status message that does not involve the STATUS_CODES
object and works for both http and https: the statusMessage
property of the response
object.
console.log("Error fetching data for user " + username + ": " + response.statusMessage);
The console output looks like this:
$ node app.js
GET https://teamtreehouse.com/sebroeder42.json: 404
Error fetching data for user sebroeder42: Not Found

Patrick O'Dacre
15,471 PointsThis is a nice solution; it eliminates the need to require('http') for just that one status message.
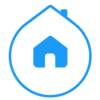
renthunt
5,419 Points@saadkhanmalik : https is not "absorbing" from http in this case. The call http.STATUS_CODES is separate from your .get request. Hope this helps.
Saad Khan Malik
25,199 PointsSaad Khan Malik
25,199 Pointsthanks, Required the http module.
But what I don't get is how the .get works on https, but "absorbs from" http. If you can help explain that one bit...
FYI the error check on the code is working fine.