Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial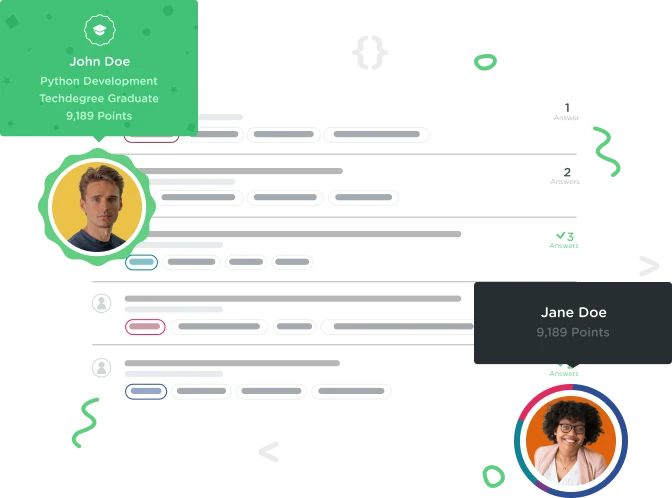

A F
1,094 PointsProperty Observer Code Challenge
I keep getting the error "error: expression pattern of type 'Bool' cannot match values of type 'Double' " when using the code below. I saw people solve this challenge using if/else statements but I thought a switch statement would be a way to make my code easier to read. How can I solve this code challenge while still using a switch statement?
class TemperatureController: UIViewController {
var temperature: Double {
didSet {
switch temperature {
case temperature > 80.0: view.backgroundColor = UIColor.redColor()
case temperature < 40.0: view.backgroundColor = UIColor.blueColor()
default: view.backgroundColor = UIColor.greenColor()
}
}
}
init(temperature: Double) {
self.temperature = temperature
super.init()
}
override func viewDidLoad() {
view.backgroundColor = UIColor.whiteColor()
}
}
1 Answer

Matte Edens
9,788 Pointsswitch
is just like an advanced set of if
statements. Deconstruct the switch
statement into an if
statement to see the problem. Even just recreating the first case should be enough. Here's how it'd break down.
if temperature = temperature > 80.0 {
}
since temperature
is a Double and temperature > 80.0
return a Bool the comparison blows up since you can't compare Bool and Double, apples and oranges.
easy fix is to say…
switch true {
case temperature > 80.0
}
Now the if
statement would be …
if true = temperature > 80.0 {
}
Comparing a Bool to a Bool. Setting the initial switch
value to true is not something I see a lot of tutorials doing, but I learned it from the Ruby community where it's common to set a value in the switch statement and compare against case values that are ranges, booleans, etc. I've done it in PHP too, and wouldn't be surprised if most languages out there allow this.