Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial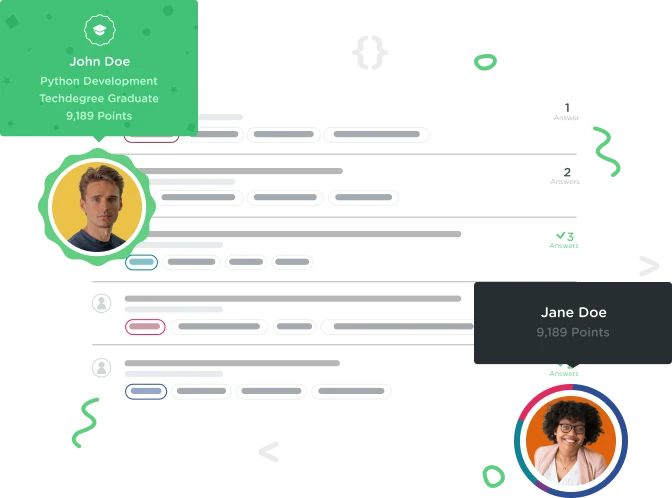

Robert Walker
17,146 PointsProtect user passwords during signup and login.
I have a sign up form that sends all the information to a signup processing script:
Sign up page -> sign up process page -> login page if successful or back to the sign up page if unsuccessful.
My worry here is that their password is exposed while sending this information from page to page, if so how do I protect their passwords?
3 Answers

Eric Butler
33,512 PointsHey Robert, This is the exact reason for using a secure (SSL) connection. Sign-up pages (and log-in pages, and any page that deals with sensitive information) should use SSL. Doing so means that no one can "sniff" the information that is sent from the sign-up page to your server. You'd make that happen by buying and installing an SSL certificate on your site, and making sure that whenever someone visits your sign-up page, they use the https:// protocol.
Now, re: sending that information page-to-page: you shouldn't keep sending the info at all! It should be sent once, on the signup page, and then you switch to a persistent session value. Here's the basic idea:
- User fills out your form on signup.php, clicks "submit."
- The form inputs are sent to the processing script on your server (signup_process.php), which validates that all fields are complete and valid, then (if the data is good) executes a database (SQL) query to add that person's info to your database. (Side-point: Your processing script should also "hash" the submitted password and store that hash in the database instead of the actual password. If you need more info about this, let me know.)
- At the end of that processing script, some kind of "isLoggedIn" variable should be stored in your site's $_SESSION scope. Something like:
$_SESSION['isLoggedIn'] = 1;
$_SESSION['user_id'] = 123456; // this value should be generated dynamically
The "user_id" would be set to whatever the user's ID is. If you're using MySQLi, you could get that value automatically with mysqli_insert_id(). If you're using PDO, you could use lastInsertId().
Note: You have to initialize sessions before you can use them. At the top of **every* page that needs to pull data from a session (in other words, at the top of every page in your site) you need to call:*
session_start();
...and it needs to be called before absolutely anything that gets output to the browser (even white space).
Then you can just pass that session value page to page, and validate/contextualize pages based on this persistent session value, instead of against the submitted username and password, over and over page to page. Doing this is much safer (although not perfectly safe, since there is such a thing as session hijacking -- pretty advanced hacking), but at least this will protect people's login information. I should also add that this is how most sites actually do handle logins and sign-ups, so I'm not just saying how I do things.
Final tip: You don't have to use https:// on every page in your site, just the ones that send sensitive information. So /signup.php should use SSL, but /index.php doesn't need to (unless there's a sign-in form on it).
Hope that gives you plenty to work with!
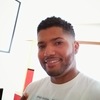
Emmanuel Salom
Courses Plus Student 8,320 PointsWhy not logging the user in After registration. Save the data to mysql and hash the password.

Robert Walker
17,146 PointsThanks again Eric!
Robert Walker
17,146 PointsRobert Walker
17,146 PointsThank you for the reply Eric, so I assume I would need to do this for all pages like reset password and so on too?
I am using a salt and crypt its just non of that matters if people can just pick it before its hashed.
Is there a particular place I should get this SSL?, I don't want to pay with my soul type of thing the site is not very big. Just wondering if there are standard places to get it from or if Godaddy and those for 3.99 are actually worth getting.
Robert Walker
17,146 PointsRobert Walker
17,146 PointsThank you Marcus too!
Eric Butler
33,512 PointsEric Butler
33,512 PointsRobert, on the page where the user actually re-creates his/her password, yes, you'd want that to be a secure page too (so like https://www.example.com/reset-password.php). The way I do password resets is with a few pages:
Re: where to get SSL certs, I'm not too smart about who's got the best stuff, I just get them from my domain registrar, since it's usually the easiest route for setup. I kinda like Namecheap, and you can see their SSL options here.
Anyway, good luck! Let me know if anything's unclear or faulty.