Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial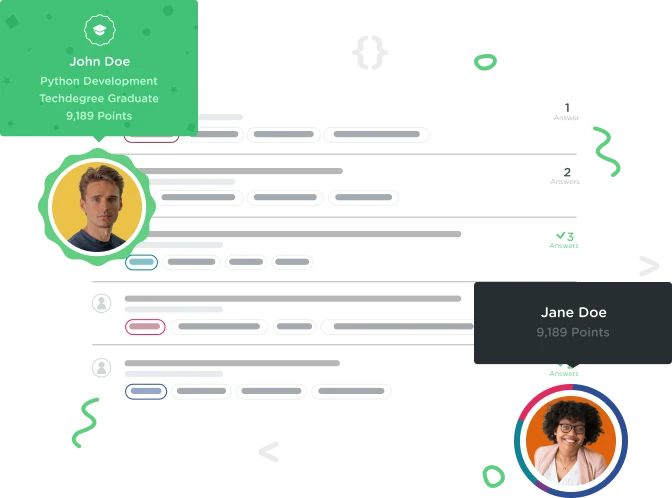

Addison Francisco
9,561 PointsProtocol Oriented Programming Part 2: The pasted code for Point struct is conflicting with the Enemy class
Still getting the hang of OOP and wanted some clarification. In the video, Pasan pastes some code from a previous course "Object-Oriented Swift" that declares a struct named Point
struct Point {
let x: Int
let y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
func surroundingPoints(withRange range: Int = 1) -> [Point] {
var results: [Point] = []
for xCoord in (x-range)...(x+range) {
for yCoord in (y-range)...(y+range) {
let coordinatePoint = Point(x: xCoord, y: yCoord)
results.append(coordinatePoint)
}
}
return results
}
}
Point
's x
and y
properties are declared as constants in that previous course, but in this video we declare a protocol with a property of type Point
that is gettable and settable.
protocol PlayerType {
var position: Point { get set }
var life: Int { get set }
init(point: Point)
}
Since Enemy
adopts the PlayerType
protocol This is causing an error in the switch statement in Enemy
class's move()
method that says Left side of mutating operator isn't mutable: 'y' is a 'let' constant
func move(direction: Direction, distance: Int) {
switch direction {
case .Up: position.y += 1
case .Down: position.y -= 1
case .Left: position.x -= 1
case .Right: position.x += 1
}
}
I came to the conclusion that I just needed to modify the Point
struct's stored properties to be variables since we are defining a protocol that declares the property requirements of Point
should be gettable and settable. Am I missing anything here?
2 Answers

JT Keller
12,731 PointsAddison - I used the same approach in regards to refactoring the constants as variables.
Also I'd like to note that var parameters are being deprecated as of Swift 3.0, so I addressed this issue for the attach function by using shadowing as seen below.
If needed here's the information related to the change: https://github.com/apple/swift-evolution/blob/master/proposals/0003-remove-var-parameters.md
func attack(player: PlayerType) {
var player = player
player.life = player.life - strength
}
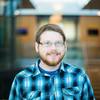
John Carty
6,444 PointsYou actually need to modify the Point that is member of the Player class.
Your method should look something like:
case "Up": position = Point(position.x+1, position.y)
syntax may be a bit off, I am coding from memory without access to Xcode.

Addison Francisco
9,561 PointsI actually downloaded the Project File and saw that he did what I did as well. Seems like an oversight.
struct Point {
// Code in the video that was causing errors
// let x: Int
// let y: Int
// Code in the Project File that resolved errors
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
func surroundingPoints(withRange range: Int = 1) -> [Point] {
var results: [Point] = []
for xCoord in (x-range)...(x+range) {
for yCoord in (y-range)...(y+range) {
let coordinatePoint = Point(x: xCoord, y: yCoord)
results.append(coordinatePoint)
}
}
return results
}
}