Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial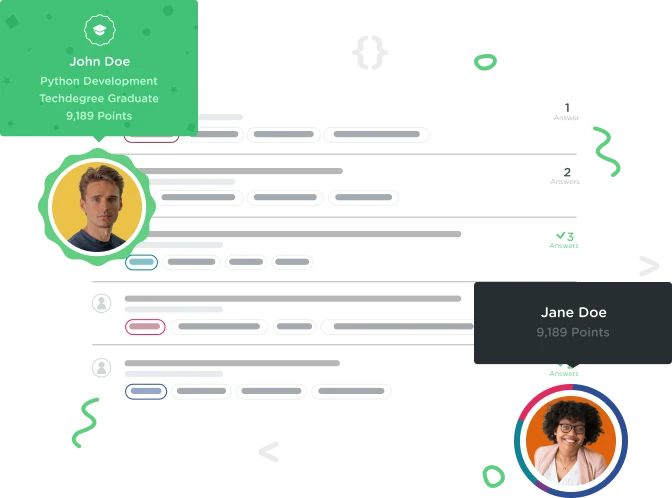

vincent batteast
51,094 Pointspublic method in the NumberAnalysis class called NumbersGreaterThanFive that returns an IEnumerable<int>.
NumberAnalysis.cs(16,49): error CS0103: The name `number' does not exist in the current context Compilation failed: 1 error(s), 0 warnings
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class NumberAnalysis
{
private List<int> _numbers;
public NumberAnalysis()
{
_numbers = new List<int> { 2, 4, 6, 8, 10 };
}
public IEnumerable<int> NumbersGreaterThanFive()
{
var NumbersGreaterThanFive = from _numbers in number
where _numbers > 5 select _numbers;
return NumbersGreaterThanFive;
}
}
}
3 Answers
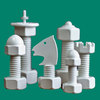
Steven Parker
231,275 PointsYou probably just have your terms exchanged.
Your code looks good except that where I would expect to see the list field "_numbers
", you have "number
" (and thus the error). And in the places (3 of them) where I would expect to see the name of a temporary variable (such as "number"), you have the name of the list field.

vincent batteast
51,094 Pointsnew error
NumberAnalysis.cs(18,12): error CS0266: Cannot implicitly convert type System.Collections.Generic.IEnumerable>' to
System.Collections.Generic.IEnumerable'. An explicit conversion exists (are you missing a cast?)
Compilation failed: 1 error(s), 0 warnings
code
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class NumberAnalysis
{
private List<int> _numbers;
public NumberAnalysis()
{
_numbers = new List<int> { 2, 4, 6, 8, 10 };
}
public IEnumerable<int> NumbersGreaterThanFive()
{
var NumbersGreaterThanFive = from number in _numbers
where number > 5 select _numbers;
return NumbersGreaterThanFive;
}
}
}

Jon Wood
9,884 PointsIn your select
you're selecting the IEnumerable
. Do select number
instead.
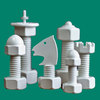
Steven Parker
231,275 Points where number > 5 select _numbers;
You got the other 2, but you forgot to change that last "_numbers
" to "number
".

vincent batteast
51,094 Pointsthank you!