Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial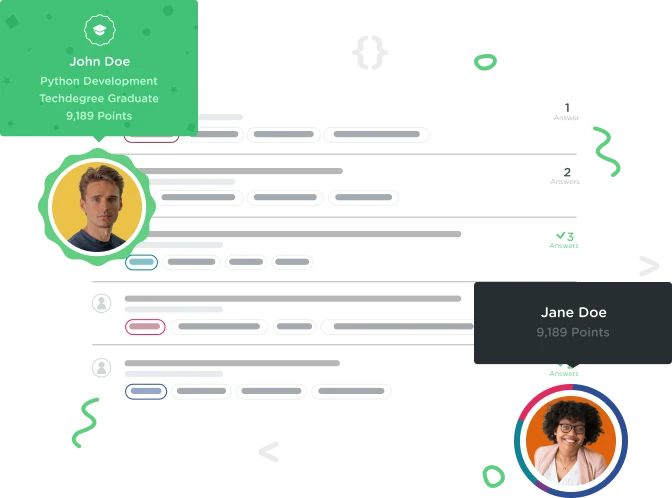

vincent batteast
51,094 Pointspulling data in C#
I have a csv file with 3 columns of random data. what I do know that each row as a phone and a fax number. I want to pull the number and aline with that row. there what I have
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace gettingdata
{
class Program
{
static void Main(string[] args)
{
string[] phone;
string[] faxnumber;
string[] name;
String fax = "C:\\Users\\vinbat\\Desktop\\fax.csv";
String[] allLines = System.IO.File.ReadAllLines(fax);
foreach(line in allLines)
{
}
}
}
}
nothing is uniform so I may have to search a couple different ways. put if you have a website or ideal on how to tackle this problem I would appreciate it
3 Answers

Samuel Ferree
31,722 PointsThere are libraries that will help you parse a csv file in C#, there are some suggestions in this stack overflow thread: https://stackoverflow.com/questions/2081418/parsing-csv-files-in-c-with-header
Can you describe your problem in more detail?
It sounds like you want to be able to select rows, by the phone number that it's in that row (but you may not know which column it it's in), so maybe you'd want to parse each row, and store the data in a Dictionary<string,string> with the phone number as the key?
The more you tell me the more specific I can be.

vincent batteast
51,094 Pointsyes I have the csv file and I can open it in excel and do stuff to the file there. But the data is the data.
more about the problem at the end I need name + fax +phone + the 3 old fields
the three fields that I need to pars could be in any of the three fields.
it could say fax (xxx)xxx-xxxx or fax: xxx-xxx-xxxx or the word fax in one column and the number in the next. but fax would be the last word in one column and the number would be the 1st thing in the next column or some other way or variation of this. but I need to pull that fax number out. plus I'm going to have to do the same to the phone number and name

vincent batteast
51,094 Pointsyes I have the csv file and I can open it in excel and do stuff to the file there. But the data is the data.
more about the problem at the end I need name + fax +phone + the 3 old fields
the three fields that I need to pars could be in any of the three fields.
it could say fax (xxx)xxx-xxxx or fax: xxx-xxx-xxxx or the word fax in one column and the number in the next. but fax would be the last word in one column and the number would be the 1st thing in the next column or some other way or variation of this. but I need to pull that fax number out. plus I'm going to have to do the same to the phone number and name.

Samuel Ferree
31,722 PointsYou need to figure out all the different ways the data can be formatted. Then you have to detect which format the data is in, and parse out the information you need.

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsFor the fax number, you could try removing all whitespace and non-numeric characters. That will leave you with just the numbers. C# has the Regex.Replace
method that you can see below:
https://msdn.microsoft.com/en-us/library/system.text.regularexpressions.regex.replace(v=vs.110).aspx
However, I would think the formatting of the data could be normalized in either the database query that produces fax.csv and/or by the front end application that users enter this data to. This just seems like a lot of work that you shouldn't have to do if the proper validation was put in place.

vincent batteast
51,094 Points for(int i= 0; i > allLines.Length; i++)
{
// allLines[i].LastIndexOf("fax: ")
int start = allLines[i].LastIndexOf("fax:");
int end = start + 13;
faxnumber[i] = allLines.Substring(start, end);
Console.WriteLine(faxnumber);
}
when I try to use the substring() I'm getting a error
CS1061 C# 'string[]' does not contain a definition for 'Substring' and no extension method 'Substring' accepting a first argument of type 'string[]' could be found #are you missing a using directive or an assembly reference?
I'm look in the that site Chris. and I just would like to thank Chris and Samuel for all your help.
any help on how to use this substring or how to put this in a if statement because I think I'm going to need to search more than one way.

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsNo problem, Vincent! allLines
is a string array, not a string. So, you'll want to call substring
on an item in the allLines
array, like this below.
Also, you'll want to change the greater than operator to less than or your loop will not iterate because 0 is not greater than the length of allLines
, so the loop will never happen.
for(int i= 0; i < allLines.Length; i++) // notice how the greater than operator changed to less than.
{
// allLines[i].LastIndexOf("fax: ")
int start = allLines[i].LastIndexOf("fax:");
int end = start + 13;
faxnumber[i] = allLines[i].Substring(start, end); // notice how I used the variable "i" to get the item.
Console.WriteLine(faxnumber);
}
I hope that helps some! Hopefully the Regex.Replace
method can help some, too, if substring
doesn't catch all the text.
Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsChris Jones
Java Web Development Techdegree Graduate 23,933 PointsDo you have any control over the creation of fax.csv?