Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial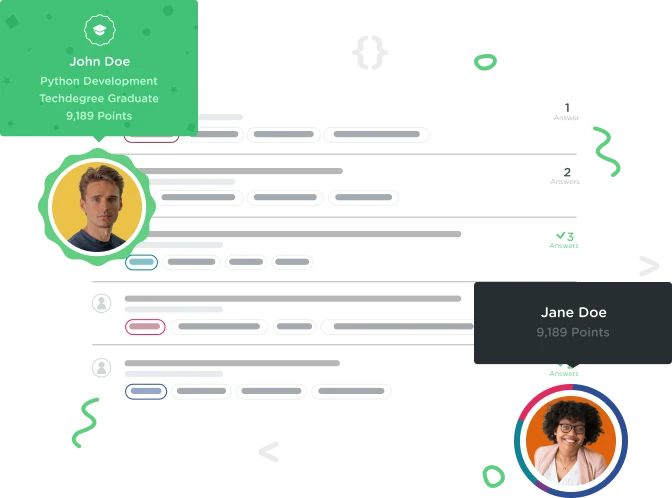

Ranvir Sahota
9,844 PointsPurpose of declaring method reutrn type
Why is is neccssary to state what will be returned in a method? Even when you return nothing you must type void. Is this for effciency in reducing memory and increasing speed?
3 Answers

Samuel Ferree
31,722 PointsIt's done because Java is a strongly typed language.
The compiler needs to know the type a function returns, when compiling your code, so it can catch type errors.
consider the following javascript
function add5(x) {
return x + 5;
}
console.log(add5("5")); //logs '55'
The code looks okay at first glance, but the user passes in a string, and in javascript, 5 is concanted to the end of the string, instead of adding 5 and 5 to get 10. The equivalent code in java wouldn't even compile
public int add5(int x) {
return x + 5;
}
System.out.println(add5("5")); //Compilation error
Now, this fails because the type of the parameter is wrong, and not the return type... but you can see how typing is used to catch errors during compilation. This strong typing also helps IDE's provide better intellisense when coding.

Samuel Ferree
31,722 PointsThere really isn't a single solid definition of strongly typed, however. any decent definition almost surely would include JAVA.
Here's the one I found that will work in most cases:
A strongly-typed programming language is one in which each type of data (such as integer, character, hexadecimal, packed decimal, and so forth) is predefined as part of the programming language and all constants or variables defined for a given program must be described with one of the data types.
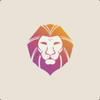
Oliver Michaelsen
Courses Plus Student 1,166 PointsIt depends on what your method does. For example, lets say you have a program that gets the users age. First, you'd want to get the users age and you can only do that if your method returns the age. Let's say you create the method public void getAge() and take it into use with your chosen object, you won't be able to get the age because it doesn't return the value, so using void here wouldn't work out. You can use void on methods that doesn't need to return anything, and example could be:
public void setAge(int newAge) {
age = newAge;
}
Anymore questions, let me or the others know :)
Ranvir Sahota
9,844 PointsRanvir Sahota
9,844 Pointscan you tell how you define a strongly typed languaage becuase I searched a few posts and it basically states there isnt an agreement on how you define a strongly tpyed langauge.
Ranvir Sahota
9,844 PointsRanvir Sahota
9,844 PointsOk thanks I'm getting closer to understanding this but I still have two questions. How does help the compiler catch type errors and how does it help IDE with intellisense? Sorry to ask so many questions.
Samuel Ferree
31,722 PointsSamuel Ferree
31,722 PointsSure, so because the compiler knows what data type the function expects, and what the type is of the variable you actually passed in, it can detect and inform the user of a type error.
Intellisense, is a sort of "precompile" that the IDE does to learn about the code, so that it can offer better suggestions.
Instead of getting an ugly type error at compile-time, your ide will give you red lines under text, similar to mispelling a word would.