Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial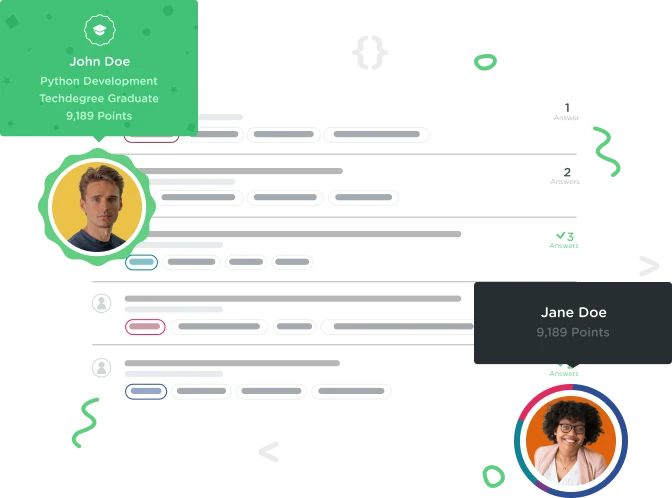

Tim Kotowski
1,655 PointsPurpose of __init__ in Classes
Hi,
I do not understand why init is used in classes. You can define a general attribute like the below example, right?
class Example: name = "Your name"
You could also write the code as:
class Example: def init(self, name): self.name = name
I understand that init is useful for running an unspecified number of arguments, such as in *args or **kwargs. Is it just another way to assign initial attributes, with the added benefit of being able to assign additional attributes later through *args and **kwargs? Or is there something I am missing?
2 Answers
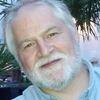
Jeff Muday
Treehouse Moderator 28,720 PointsI am glad you are thinking about the "meta" design of Python classes. You will use these every day as a programmer so it is good to have a deeper understanding about what is going on.
One of the things I love about Python (and for that matter all programming languages) is that there is no single right way to do things. You are correct in your assumption that even if __init__()
did not exist, you could create other ways of instantiating the properties of an object.
Often, you will use classes that have "Factory Methods," that is, a method that can create/instantiate new objects (e.g. copies or variations of its own class). Though ultimately, factory methods will call the class __init__()
as a last step.
Why we have __init__()
- To provide constructor compatibility across the Python language. Python considers objects to be "first class citizens" thus every object can be passed to functions, checked for equality, and used in computations and assigment statements. Some of this would not be easily done unless there were a standard "constructor" method.
- To provide design compatibility and interoperability with non-Python APIs.
A number of important Python libraries are optimized for speed using lower level languages like 'C' language. The standard constructor definition makes it possible to easily interoperate with these with no special tricks or names.
- To allow uniform class inheritance all the way down to "native" data types like sets, lists, dictionaries, etc.
I can overide __init__()
when making a user defined class or omit writing an __init__()
of a child object, and expect to use the parent's __init__()
method. And if the parent's init doesn't exist, I can use the parent's parent -- all the way down to the "base object". I also have the ability reference the "parent" or super class methods and not have to provide any special instantiation.

Tim Kotowski
1,655 PointsThank you for the time to answer, I appreciate it. I see now that this is something that is helpful in overriding attributes and for inheritance.
I do want to give a bit of advice to Treehouse, if you will consider it.
Frankly, I think that the intro to OOP course that Treehouse has for Python needs some revamping. There are a lot of concepts that are not clearly pointed out.
For example, the format for writing an attribute from an instance (instance_name.attribute_name) is not clearly explained. The vocabulary is kind of explained, but the syntax is not. That held me back for a week alone.
When I tutor Spanish, the first thing I do is to check what my student is weak in. Is he or she weak in vocabulary, or grammar? Once I pinpoint the problem, I assign them to memorize the areas where they are weak.
The truth is that memorization is a base component of language learning. You need a basic understanding of vocabulary and grammar to be able experiment with the language and construct more complex ideas, or in this case, code.
Unless I am mistaken, Treehouse lacks a resource like a cheat sheet or an interactive flashcard set to help people study these basic language building blocks.
From my own experience, it is easier to give advice than to implement it. But I believe that a simple feature like that would greatly help your students.
Sincerely,
Tim
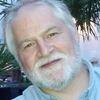
Jeff Muday
Treehouse Moderator 28,720 PointsThanks for taking the time to write such an authentic/meaningful response!
I really like your idea of building out some summary "cheat sheets".
I am not directly connected to planning the curriculum, but I am pretty sure there are plans to refresh some of the material. Kenneth Love did a great job and I am a big fan of his, but there are some opportunities to break it up into more "bite-sized" chunks.
I would probably remove the lesson about "attribute" classes, though Kenneth is kind of setting us up to handle studying intermediate/advanced Django and Flask concepts-- in particular "mixins".
https://docs.djangoproject.com/en/3.1/topics/class-based-views/mixins/