Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial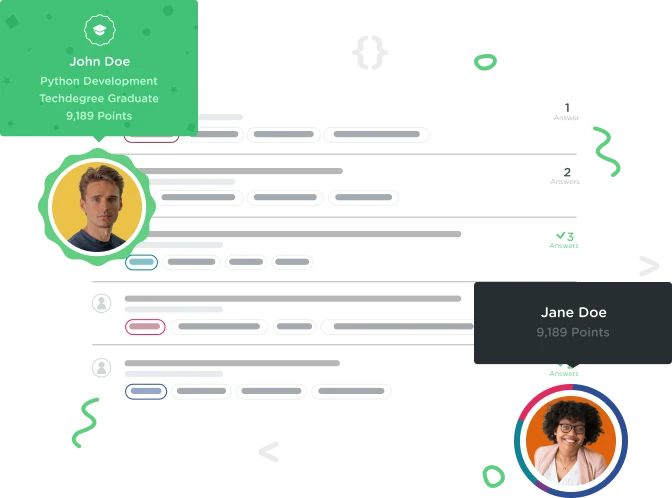

Roland Cedo
21,261 PointsPushing to todo_list_item array while it is an attr_reader?
It was to my understanding that attr_reader allows us to read an instance variable, but not write to it?
In this video, we create an instance variable called @todo_items and set it as an attr_reader:
class ToDoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = Array.new()
end
end
How are we able to execute the following code if we were only able to read todo_items?
def add_item(name)
todo_items.push(TodoItem.new(name))
end
1 Answer
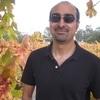
Kourosh Raeen
23,733 PointsYou don't need write access to push items into the todo_items array. You could even do that outside the class. Try adding this line:
todo_list.todo_items.push("Bread")
Here's the complete code:
require "./todo_item"
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
end
todo_list = TodoList.new("Groceries")
todo_list.add_item("Milk")
todo_list.add_item("Eggs")
todo_list.todo_items.push("Bread")
puts todo_list.inspect
What wouldn't work is assigning something to todo_items, like a new empty array as in:
require "./todo_item"
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
end
todo_list = TodoList.new("Groceries")
todo_list.add_item("Milk")
todo_list.add_item("Eggs")
todo_list.todo_items.push("Bread")
todo_list.todo_items = []
puts todo_list.inspect
You get an error saying : undefined method 'todo_items='
To fix that add an attr_writer:
require "./todo_item"
class TodoList
attr_reader :name, :todo_items
attr_writer :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
end
todo_list = TodoList.new("Groceries")
todo_list.add_item("Milk")
todo_list.add_item("Eggs")
todo_list.todo_items.push("Bread")
todo_list.todo_items = []
puts todo_list.inspect
Now it works and you can see that the three items in the array are gone since you recreated an empty array.
Roland Cedo
21,261 PointsRoland Cedo
21,261 PointsInteresting, so calling a method to modify the the contents within the array (such as push, pop, shift, etc.) isn't considered "writing" to the instance variable? I suppose my misunderstanding came from thinking that it would be. It makes sense that variable assignment wouldn't be allowed in an attr_reader situation though.
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsThat's correct. To be able to assign to an instance variable outside the class you need to either use attr_writer or define the setter method yourself.
Roland Cedo
21,261 PointsRoland Cedo
21,261 PointsThat answers it, thanks for knowledge drop Kourosh!