Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial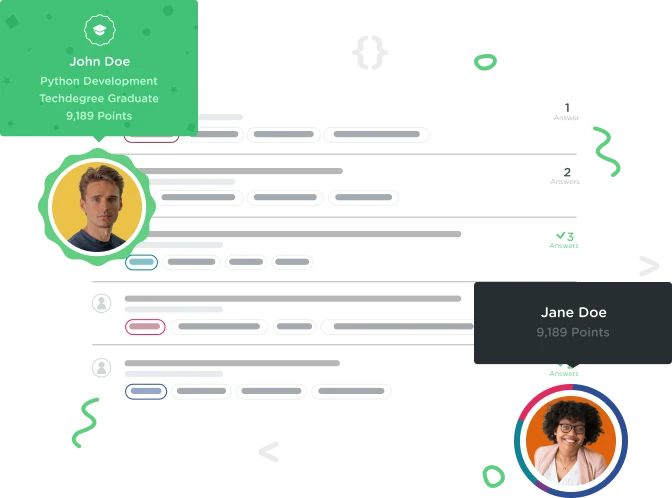

Faddah Wolf
12,811 Pointsput in correct answer, works in my code editor, does not work in test for JavaScript Unit Testing
i have put in what i believe to be the correct answer for this coding quiz in JavaScript Unit Testing. i took my code over to a code editor and it works just fine, passed the test with mocha & chai and everything, but the test keeps saying "Bummer!" and i didn't pass. i cannot figure out why.
here is my subtraction.js ā
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!');
} else {
return number1 - number2
}
}
module.exports.subtraction = subtraction;
and here is my subtraction_spec.js ā
var expect = require('chai').expect;
describe('subtraction', function () {
var subtraction = require('../WHEREVER').subtraction;
it('only works with numbers', function () {
var num1 = 'fifteen';
var num2 = false;
var result = function() { subtraction(num1, num2); };
expect(result).to.throw(Error);
expect(result).to.throw('subtraction only works with numbers!');
});
});
of course, in my own code editor, i change require('../WHEREVER').subtraction;
to require('../game_logic/subtraction.js').subtraction;
, but that should not be that big a difference in the coding quiz.
like i said, this works just fine when i run it in my code editor with mocha test/subtraction_spec.js
, but fails in the Treehouse quiz. any help here as to what i am missing?? Guil Hernandez & Joseph Fraley ā any help you or anyone else could offer would be great. thank you in advance.
yours,
ā faddah portland, oregon, u.s.a.
var expect = require('chai').expect;
describe('subtraction', function () {
var subtraction = require('../WHEREVER').subtraction;
it('only works with numbers', function () {
var num1 = 'fifteen';
var num2 = false;
var result = function() { subtraction(num1, num2); };
expect(result).to.throw(Error);
expect(result).to.throw('subtraction only works with numbers!');
});
});
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!');
} else {
return number1 - number2
}
}
module.exports.subtraction = subtraction;

Faddah Wolf
12,811 Pointshello Ryan Zimmerman,
thank you for responding. however, i don't quite understand your pointing me towards ā
expect().to.be.a();
... as in...
expect(result).to.be.a('number');
... i don't understand why you are suggesting that, as that is not what this code challenge in the learning module is testing. in the previous videos just before this challenge, we were taught the throw
method in the chai.js API, for making sure errors are properly thrown. in fact, the text in the challenge says, quite explicitly, "Make sure to check for subtraction's specific error message!"
but ok, i can add what you suggest in there also. here's a re-vamped version subtraction_spec.js, adding in what you suggest ā
var expect = require('chai').expect;
describe('subtraction', function () {
var subtraction = require('../WHEREVER').subtraction;
it('only works with numbers', function () {
var num1 = 'fifteen';
var num2 = false;
var num3 = 15;
var num4 = 6
var result1 = function() { subtraction(num1, num2); };
var result2 = subtraction(num3, num4);
expect(result1).to.throw(Error);
expect(result1).to.throw('subtraction only works with numbers!');
expect(result2).to.be.a('number');
expect(result2).to.be.equal(9);
});
});
... so i run the above in my editor ā changing the var subtraction = require('../WHEREVER').subtraction
to var subtraction = require('../game_logic/subtraction.js').subtraction
ā with all the proper node modules, and it runs and tests and passes all just fine. again, in the code challenge on Team Treehouse, it just comes back with the "Bummer!" message. so i implemented what you said to be in there, and it works fine elsewhere, but i still cannot pass the code challenge.
any further help you, or anyone else could possibly offer, would be appreciated. Guil Hernandez and Joseph Fraley ā i would appreciate any input you have here.
best,
ā faddah portland, oregon, u.s.a.
1 Answer

Lukas Dahlberg
53,736 PointsAfter dealing with this one for far too long, the answer turned out to be irritatingly simple. They've already done the work for you; all you have to do is call the subtraction function so that the 'course' can pass the information in for you.
expect(subtraction).to.throw('subtraction only works with numbers!');

Faddah Wolf
12,811 PointsLukas Dahlberg,
thank you for responding. and yes, i came to that conclusion also ā i still included Ryan Zimmerman's suggestion of ā
expect(result).to.be.a('number');
so the section of my code in the answer was:
expect(result1).to.throw('subtraction only works with numbers!');
expect(result2).to.be.a('number');
...and that passed. but i agree, the wording of the code challenge is very awkward and doesn't really describe the brief statement they are looking for very well. oh well, passed this one. onward. thank you for the help.
best,
ā faddah portland, oregon, u.s.a.
Ryan Zimmerman
3,854 PointsRyan Zimmerman
3,854 PointsLook up in Chai
expect().to.be.a();