Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial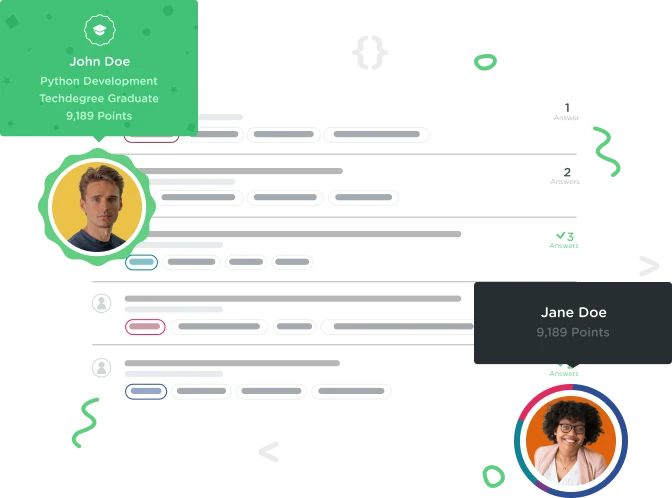

Eldar Burnashev
1,836 PointsPython Basic, Functions, challenge 2 of 2
Hello! I'm stuck. What's wrong with this code?
def add_list(list):
return sum(list)
def summarize(list):
total = sum(list)
words = ("The sum of the {} is {}.".format(list,total)
3 Answers

Stone Preston
42,016 Pointsyou are very close. you have a few minor things wrong with your code.
you need to remain consistent with your indentation. (you used 3 spaces the first function and 4 the next).
you also dont need the parenthesis at the start of your words variable (you have an opening one at the start but its missing its matching closing parenthesis, so either remove the first one or add another to the end).
your string is incorrect. it needs to be "The sum of {} is {}." not "The sum of the {} is {}.". so remove the word the.
you also need to return your words variable at the end.
its also probably not a good idea to use list as a paramater name since list is a function name. I would change it to something else to avoid any sort of confusion
see below
def add_list(some_list):
return sum(some_list)
def summarize(some_list):
total = sum(some_list)
words = "The sum of {} is {}.".format(some_list,total)
return words
technically I think they want you to use the add_list function you created first instead of sum(), so this would also work:
def add_list(some_list):
return sum(some_list)
def summarize(some_list):
total = add_list(some_list)
words = "The sum of {} is {}.".format(some_list,total)
return words
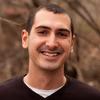
tihomirvelev
14,109 PointsHello,
It the last line of code I don't see the number between the curly brackets. You have to specify the order in which the format method put the variables.
Try it like that:
words = ("The sum of the {0} is {1}.".format(list,total)
Hope that helps. Good luck!

Stone Preston
42,016 Pointsadding the order is not necessary, it will work either way

Eldar Burnashev
1,836 PointsThank you, but local workspace thinks that promblem is somewhere else(
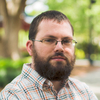
Kenneth Love
Treehouse Guest TeacherAdding the index position is only required in Python 2.6 and below.
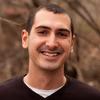
tihomirvelev
14,109 PointsTry to change the "list" parameter in the functions with something else. For example "arg".
list is reserved function in python and I think this is the real problem. It is making a problem in my machine anyway. :)

Eldar Burnashev
1,836 PointsProblem already solved, thank you.)
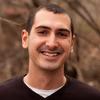
tihomirvelev
14,109 PointsGreat. That was a bad answer anyway. It actually worked with "list" parameter after further testing. :)

Stone Preston
42,016 Pointsits still probably a good idea not to use list as a name. it does work, but I would use a different name to avoid confusion. I edited my answer to include this as its a valid point.
Eldar Burnashev
1,836 PointsEldar Burnashev
1,836 PointsThank you! It's actually helps! Definitely I should be more attentive.