Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial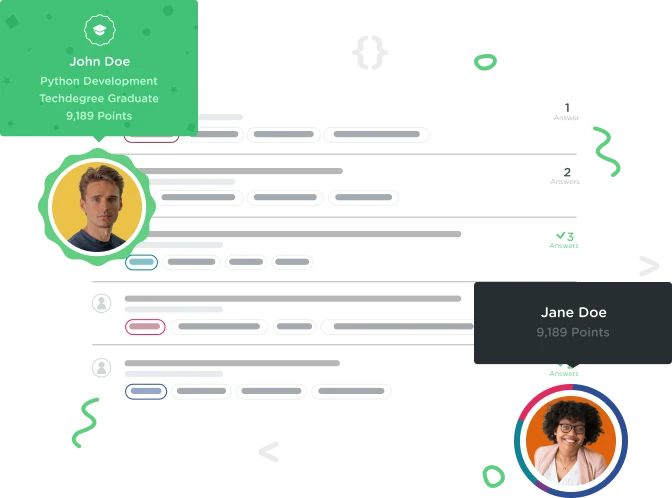

Nicholas Anstis
2,095 PointsPython Basic last challange
I can't figure it out and it'd be nice to have a proper example this time. I've already asked for help but the hints didn't help me a lot :P
Thanks in advance
Question : Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1.
import random
num = random.randint(1, 99)
start = 5
guess = int
def even_odd(num):
if guess == secret:
print("{} is even".format(num)
else:
print("{} is odd".format(num)
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while len(start) False:
else:
if start == False:
break
3 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsOkay so you have a few problems here.
First, you don't need to change the definition of the even_odd
function, so don't put any code in that block.
Second, you need to set the value of num
inside the loop using the random.randint
method so it will use a different value for each iteration.
Third, when it says to "Make a while
loop that runs until start
is falsey.' it just means:
while start:
# loop code goes here
Because you have to decrement start
inside the loop, it will eventually reach zero and that is considered 'falsey', at which point the loop will stop.
Fourth, you need to use the even_odd
function inside the loop and as part of an if
conditional. e.g.
if even_odd(num):
# code to run if num is even
else:
# code to run if num is odd
Fifth, you don't need guess
or secret
.
And lastly, be careful that you are consistent with your indentation. Use either 2 or 4 spaces for each level of indentation, where possible. I think at some point Kenneth talks about the PEP 8 style guide/convention, which helps to keep your Python code consistent and clean.

Iain Simmons
Treehouse Moderator 32,305 PointsI didn't really want to just give you the answer, but here it is:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
num = random.randint(1, 99)
if even_odd(num):
print('{} is even'.format(num))
else:
print('{} is odd'.format(num))
start -= 1

Nicholas Anstis
2,095 PointsYour first answer got me really close thank you a lot :D
I understood my errors

Iain Simmons
Treehouse Moderator 32,305 PointsIf my answer helped, please mark it as 'best answer'. :)

Nicholas Anstis
2,095 PointsI can't get it right it says task one is no longer passing. It would be nice to have a proper example like the actual code so I can see what i'm doing wrong because right now i have no idea i've checked my indentations and all but luck's not on me today :P So please if anyone could give me an example that'd be very nice :D!
Nicholas Anstis
2,095 PointsNicholas Anstis
2,095 PointsOk thanks a lot but how do I decrement Start that was originaly equal to 5 by 1?
"Finally, decrement start by 1."
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsJust set
start
equal to the value ofstart
minus 1:start = start - 1
Or with the shorthand form:
start -= 1