Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial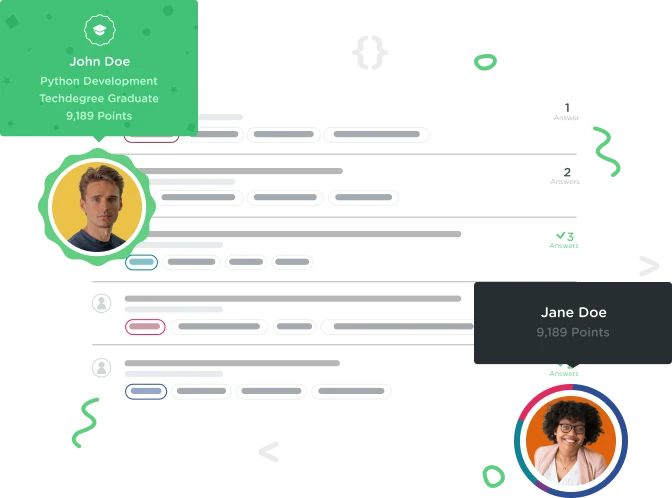
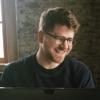
Pepijn Dekker
Courses Plus Student 5,231 PointsPython basics challenge question - Where did I go wrong?
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
if int(num):
print(int(num) ** 2)
except ValueError:
print(num * len(num))
squared(5)
squared("2")
squared("tim")
2 Answers
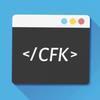
Aayush Mitra
24,904 PointsHello Pepijn,
You have the general idea of what to do. Your code worked in the code editor. You just have a few errors.
I added numbers to the lines of code so you can understand it better.
Here is your code:
1 # EXAMPLES 2 # squared(5) would return 25 3 # squared("2") would return 4 4 # squared("tim") would return "timtimtim" 5 def squared(num): 6 try: 7 if int(num): 8 print(int(num) ** 2) 9 except ValueError: 10 print(num * len(num)) 11 12 squared(5) 13 squared("2") 14 squared("tim")
First of all, on lines 8 and 10 you wrote the function print. The challenge does not want you to print, they just want you to return so change that to return.
Now your code should look like this:
EXAMPLES
squared(5) would return 25
squared("2") would return 4
squared("tim") would return "timtimtim"
def squared(num): try: if int(num): return int(num) ** 2 except ValueError: return num * len(num)
squared(5) squared("2") squared("tim")
Next, you don't want to use an if statement for this. What would be easier would be to put the number into a variable. Like this:
num = int(num)
Lastly, the challenge is not looking for a specific error. delete the ValueError after except on line 9.
After doing all of these steps, the code should work. Here is the final code:
def squared(num): try: num = int(num) return num ** 2 except: return num * len(num)
squared(5) squared("2") squared("tim")
If you have anymore questions on how this works, or if this does not pass please tell me.
I hope this worked for you!
Thanks!
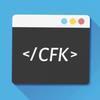
Aayush Mitra
24,904 PointsSorry the code did not format correctly. Here it is:
def squared(num):
try:
num = int(num)
return num ** 2
except:
return num * len(num)
squared(5)
squared("2")
squared("tim")
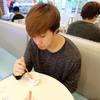
Sahapap thaesungnern
3,070 Pointsthank you! its correctly.
Pepijn Dekker
Courses Plus Student 5,231 PointsPepijn Dekker
Courses Plus Student 5,231 PointsThanks for clearing it up for me.
One last question, why wouldn't we want to use an if statement to see if num is an integer?
Aayush Mitra
24,904 PointsAayush Mitra
24,904 PointsWell you are correct, even I tried it out in the editor and I thought it was correct, which it is. It is just that treehouse does not want you to do it that way I guess. I do not know, I may actually look into that but I think since you are using the the try statement which is almost like an if statement but instead of elif you are catching an error. I guess that putting an if statement inside an almost-like if statement is kind of doing extra work, where you can just use a return statement.
I know this was kind of a weird answer, because I am a bit confused why they counted it wrong in the first place, but I hope this helps.
Thanks! :)