Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial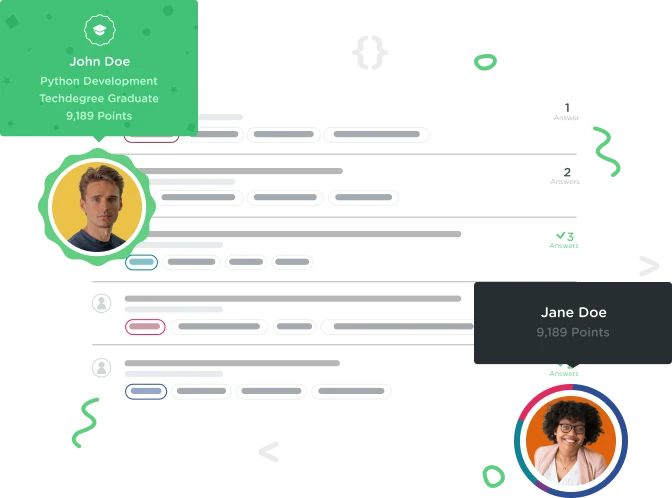

Nicholas Anstis
2,095 PointsPython Basics End challange
I can't get it right it says task one is no longer passing. It would be nice to have a proper example like the actual code so I can see what i'm doing wrong because right now i have no idea i've checked my indentations and all but luck's not on me today :P So please if anyone could give me an example that'd be very nice :D! (Already asked for help multiple times and I only got hints i'd like the actual code now please.)
1) For this challenge, we're going to need to use the random library. Add an import to the top of your file to bring it in.
2) Make a new variable, outside of the even_odd function, named start that's assigned to the number 5.
3) Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1.
Thanks in advance
import random
start = 5
while start:
num = random.randint(1, 99)
if even_odd(num):
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start = 4
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
2 Answers

Himal Shakya
20,641 PointsI removed the line after "even_odd" function and decreased start by 1, instead of setting it to 4 I was able to get this after the two changes:
# CODE STARTS HERE
import random
start = 5
while start:
num = random.randint(1, 99)
if even_odd(num):
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start = start - 1
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
# CODE ENDS HERE

Himal Shakya
20,641 PointsFor Python3, I had to move the even_odd function before using it, somehow my previous code works on Python2, I was using Python Shell and using the following command exec(open('C:/location_tomy_file/even.py').read())
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
num = random.randint(1, 99)
if even_odd(num):
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start = start - 1

Nicholas Anstis
2,095 PointsThanks a lot that is it and i understood my errors :D That was of great help :D

Freddy Venegas
1,226 PointsQuestion, am I supposed to be seeing an 5 values returned? I just ran the code sample above and I got this
19 is odd
8 is even
36 is even
20 is even
23 is odd
Thanks.
Nicholas Anstis
2,095 PointsNicholas Anstis
2,095 PointsThanks for the answer but I still get the same error that task one is no longer passing I don't know what to do :/