Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial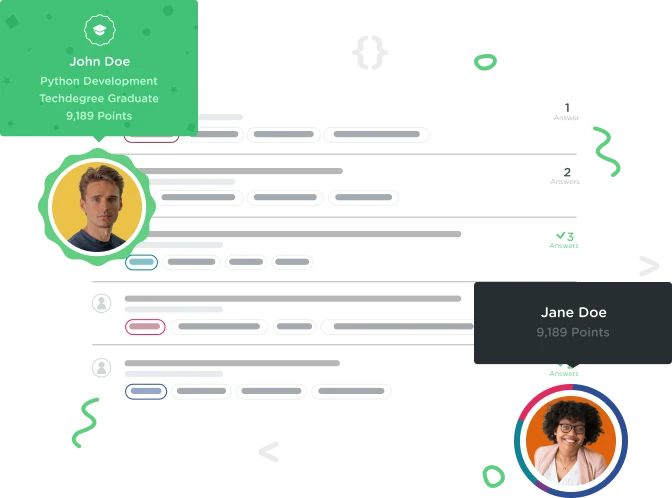
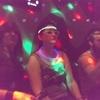
JoAnna Black
659 PointsPython Basics: Fully Functional video: how does .lower work?
I was curious what the .lower bit of code does when it was attached to an argument in the function lumberjack(). I tried taking it out but that caused an error I didn't understand. When I looked up .lower, I could only find information on how this works with strings, as in it will return a lowercase version of the string.
Any help on what this does would be cool, Kenneth doesn't really explain it in the video.
def hows_the_parrot():
print("He's pining for the fjords!")
hows_the_parrot()
def lumberjack(name, pronoun):
if name.lower() == "joanna":
print("JoAnna's a lumberjack and she's OK!")
else:
print("{} sleeps all night and {} works all day!".format(name, name))
lumberjack("Greg")
3 Answers
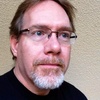
Chris Freeman
Treehouse Moderator 68,423 PointsThe .lower()
is a method on a string object. in the your code, name.lower()
says call the lower()
method on the object name
. If name
is a string it will call the inherited built-in method to return a copy of the string object name
with all the characters changed to lowercase. The object name
is not affected:
>>> foo = "STRING" # define a string object
>>> foo.lower() # call the lower() method
'string' # returns a lowercased copy of object
>>> foo
'STRING' # original string object not affected
If name
was not a string object, then an error would be raised:
>>> name = 45
>>> name.lower()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'int' object has no attribute 'lower'

Gavin Ralston
28,770 PointsJust as an aside, remember you can always call help at the python interactive line when you're not sure what a function does.
help(str.lower)
Which would have brought you to a screen like this:
Help on method_descriptor:
lower(...)
S.lower() -> string
Return a copy of the string S converted to lowercase.
That would have provided that information for you pretty clearly.
This is exactly the place you want to ask questions like this, so I'm not trying to be glib or anything. Just pointing you in the right direction to get your answer quickly.
If you weren't exactly sure what the variable was you could have done something like this, too:
name = "Joanna"
help(name.lower)
The help function there would have figured out name was a string, then given you the help information for the lower() function you called on it.
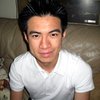
jason chan
31,009 Pointslower converts the entire string to lower case. Because python is probably a case sensitive programming language. It has to do with passwords.
Example.
a = A
if a == a:
print("true");
else:
print("false")
// stuff like HelLo would not work to lower would convert everything lower case so things would not be case sensitive for a search.
JoAnna Black
659 PointsJoAnna Black
659 PointsThanks, after stepping away from this last night and looking at it again today it makes sense why you would want to use something like this in your code, but it would have been nice if the video explained it instead of just putting it in there without really saying how that method works or what the functionality is within the example.