Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial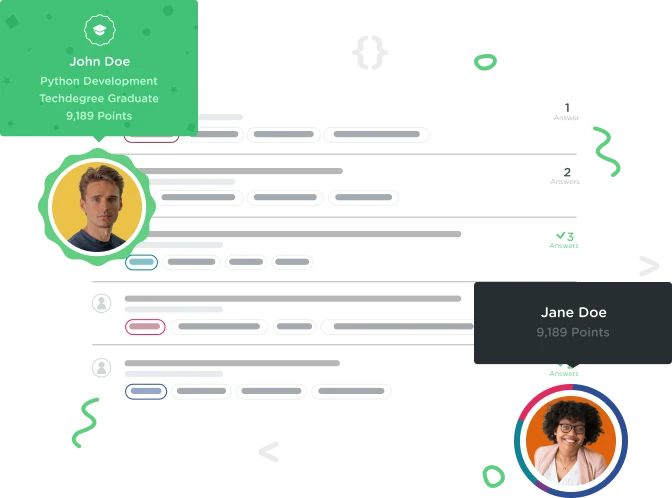

Chris Navarro
371 PointsPython Basics- functions
Hey guys, I don't understand why my Python code is not working properly. Is my syntax wrong? Can't I call another function within another function? Thank you!
So basically the first question is: Make a function named add_list that takes a list. The function should then add all of the items in the list together and return the total. Assume the list contains only numbers. You'll probably want to use a for loop. You will not need to use input().
My code:
def add_list(list):
total=sum(list)
return total
But the second one tells us to make a function summarize() that takes the list and prints "The sum of X is Y", where X is the list and Y is the sum. This is where my code does not work.
My code (below the first one):
def summarize(list):
print "The sum of {} is {}".format(list.str(), add_list(list))
4 Answers
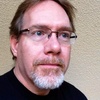
Chris Freeman
Treehouse Moderator 68,441 PointsIn the line
print "The sum of {} is {}".format(list, add_list(list))
The .str() is unnecessary. The .format()
method does the conversion to a string automatically.
The other suggestions of not using the built-in type list
as a variable name and to use a loop instead of the built-in function some are also valid but aren't causing the error.

Josh Keenan
20,315 PointsTry to avoid using 'list' as an argument name, it is a reserved word and in certain IDEs it can cause problems as it thinks you're trying to call the list() function, use my_list or list1, I stick to simple things like that normally!
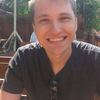
Krzysztof Kucharzyk
Front End Web Development Techdegree Student 4,005 PointsHmm I think you should do something like this:
def add_list(lst) :
result = 0
for item in lst:
result += item
return result
And for 2nd part define summarize with lst value and then return:
def summarize(lst):
return "The sum of " + str(lst) + " is " + str(add_list(lst)) + "."

Chris Navarro
371 PointsThank you so much! :)