Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial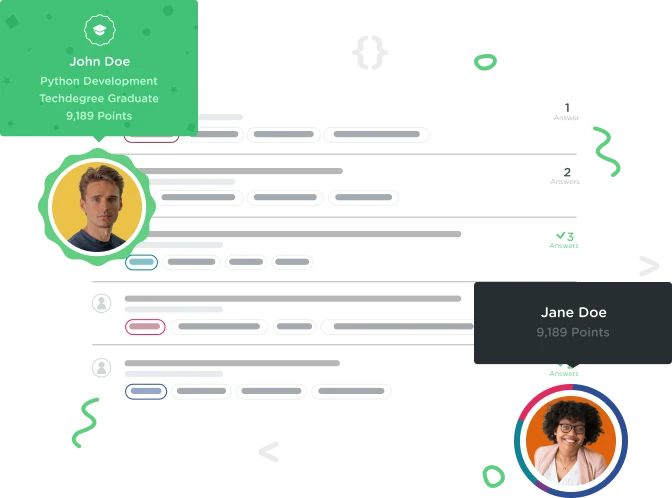

Unsubscribed User
1,747 PointsPython Basics - Refactoring lesson problem
Hi. Following along in my Workspace, I think I matched the code to Craig's, but I started to get confused when he created the function calculate_price and changed num_tickets to number_of_tickets. I couldn't find anywhere that number_of_tickets was defined, but his code worked fine.
My code resulted in this:
treehouse:~/workspace$ python masterticket.py
There are 100 tickets remaining.
What's your name? Charles
How many tickets would you like, Charles? 4
The total due is $<function calculate_price at 0x7fda9d1c8e18>.
SERVICE_CHARGE = 2
TICKET_PRICE = 10
tickets_remaining = 100
def calculate_price(number_of_tickets):
return (number_of_tickets * TICKET_PRICE) + SERVICE_CHARGE
while tickets_remaining >= 1:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What's your name? ")
num_tickets = input("How many tickets would you like, {}? ".format(name))
try:
num_tickets = int(num_tickets)
if num_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining".format(tickets_remaining))
except ValueError as err:
print("Oh no, we ran into an issue. {}. Please try again".format(err))
else:
amount_due = calculate_price(num_tickets)
print("The total due is ${}.".format(calculate_price))
should_proceed = input("Do you want to proceed? Y/N ")
if should_proceed.lower() == "y":
# TODO: Gather credit card information and process it
print("SOLD!")
tickets_remaining -= num_tickets
else:
print("Thank you anyway, {}!".format(name))
print("Sorry, the tickets are all sold out!!! :(")
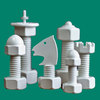
Steven Parker
231,275 PointsUse the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
And those 3 marks need to be accents ("back-ticks") instead of apostrophes.
3 Answers
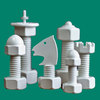
Steven Parker
231,275 PointsFrom what I can see in the unformatted code, "number_of_tickets" is a function parameter name.
Parameters only act as placeholders for variables or values that are passed when the function is called, and therefore would not be defined separately.
Also, I spotted this expression: ""The total due is ${}.".format(calculate_price)
", which doesn't make sense if "calculate_price" is the name of a function. Did you mean to call the function and pass it an argument there?

Unsubscribed User
1,747 PointsThank you Steven
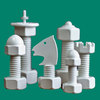
Steven Parker
231,275 PointsHappy to help. Feel free to edit and fix your formatting for posterity. Hint: if you add the 2 letters "py" immediately after the first 3 apostrophes, markdown will apply syntax coloring to make the code even more readable.

Unsubscribed User
1,747 PointsSteven - Bingo, you spotted the error. Instead of writing print("The total due is ${}.".format(amount_due)) I wrote print("The total due is ${}.".format(calculate price))
It is amazing how easy it is to get stuck in a state of blindness, not being able to see the errors that are right there. I suspect that writing code does wonders for exercising the "attention to detail" muscles. Thanks again.
Unsubscribed User
1,747 PointsUnsubscribed User
1,747 PointsSorry, I thought I followed directions on how to paste code but obviously didn't get it right.