Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial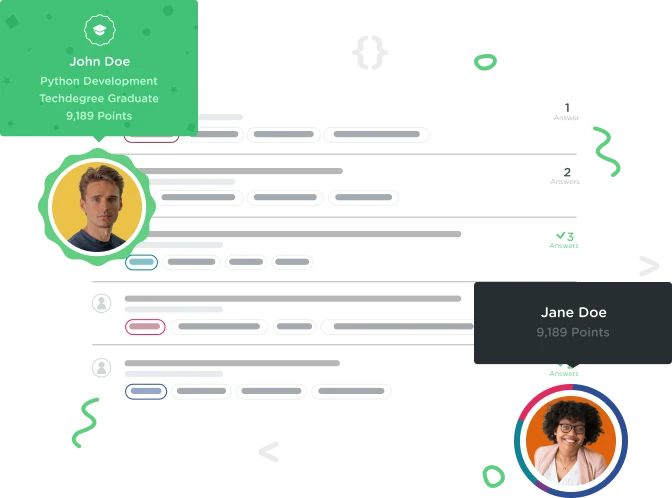

James Dunn
3,041 PointsPython Code Challenge, "Hit Points"
I've been pounding my head against this challenge for the past couple of days and finally had to admit that I'm clueless as to what I'm shooting for here.
My code should be attached already. If not I'll be happy to add it.
Simply put, what am I missing?
The instructions are as follows:
"Our game's player only has two attributes, x and y coordinates. Let's practice with a slightly different one, though. This one has x, y, and "hp", which stands for hit points.
Our move function takes this three-part tuple player and a direction tuple that's two parts, the x to move and the y (like (-1, 0) would move to the left but not up or down).
Finish the function so that if the player is being run into a wall, their hp is reduced by 5. Don't let them go past the wall. Consider the grid to be 0-9 in both directions. Don't worry about keeping their hp above 0 either."
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction):
x, y, hp = player
if direction == "LEFT":
if x < -9:
player = x, y, hp - 5
else:
player = x - 1, y, hp
if direction == "RIGHT":
if x > 9:
player = x, y, hp - 5
else:
player = x + 1, y, hp
if direction == "UP":
if y > 9:
player = x, y, hp - 5
else:
player = x, y + 1, hp
if direction == "DOWN":
if y < -9:
player = x, y, hp - 5
else:
player = x, y - 1, hp
return x, y, hp
3 Answers
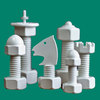
Steven Parker
231,275 PointsIt looks like you have the right idea, but the "direction" is not a string in this function. Instead, it's a tuple, as shown in the examples in the comments.
So you'll need to determine where the location might be after the direction is applied, and then test for hitting a wall.
Also, if you hit any wall, be sure to not process any other direction.
Update (Apr 10): You're getting close, but:
- you don't need any loops
- deduct hit points without changing x or y when any limit is exceeded
- the "if" line needs a colon at the end

James Dunn
3,041 PointsSorry for not replying sooner. This is my first time back in 22 days according to treehouse. Life got hectic.
Anyhoo, I've kept what you said in mind. However, I think I'm missing something that, with my luck, is obvious. Perhaps it is just a case of trouble understanding tuples.
That being said here is the code I have now. It dawned on me that I was ignoring the direction variable that is being passed. So here is my latest attempt at this.
EXAMPLES:
move((1, 1, 10), (-1, 0)) => (0, 1, 10)
move((0, 1, 10), (-1, 0)) => (0, 1, 5)
move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction): x, y, hp = player x1, y1 = direction
for d in direction:
for p in player:
if x + x1 < 0
x = 0
hp -= 5
elif x + x1 > 9:
x = 9
hp -= 5
elif y + y1 < 0:
y = 0
hp -= 5
elif y + y1 > 9:
y = 9
hp -= 5
else:
x += x1
y+= y1
return x, y, hp
It is still failing and I'm plum stumped. I really could use some fresh eyes and, obviously, the help of someone with a better grasp of tuples than I have.
Thank you.

James Dunn
3,041 PointsFinally got it. Thanks man.