Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial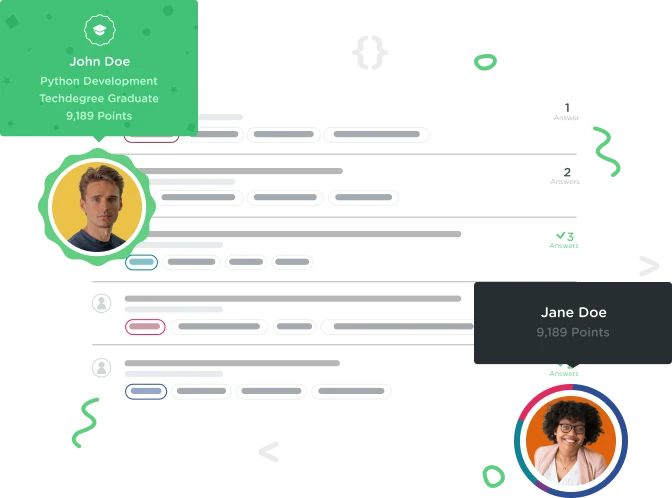
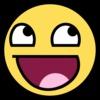
Marcus Schumacher
16,616 PointsPython code challenge, please help
Prompt: Create a function named combiner that takes a single argument, which will be a list made up of strings and numbers.
Return a single string that is a combination of all of the strings in the list and then the sum of all of the numbers. For example, with the input ["apple", 5.2, "dog", 8], combiner would return "appledog13.2". Be sure to use isinstance to solve this as I might try to trick you.
I try running this block in spyder to test.
def combiner(made_up_stuff):
my_string = ""
total = 0
for thing in made_up_stuff:
if type(thing) == str:
my_string += thing
elif (type(thing) == float or type(thing) == int) and thing != str:
total += thing
total = str(total)
my_string += str(total)
return my_string
I am getting the following error: can only concatenate str (not "int") to str
But I coerced total to a string! What am I doing wrong?
1 Answer
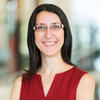
Louise St. Germain
19,424 PointsHi Marcus,
The issue is pretty minor - the two lines where you're coercing total and my_string to strings (near the end of the code) are indented one level too far in, which means they are executing during the for loop where you're trying to tally the numbers and concatenate the strings separately. This means that at the end of the first iteration of the for loop, it turns total
into a string, and then gets upset at the next iteration when you try to add a number to the now-string total
.
If you unindent those two lines (see below), it should work.
def combiner(made_up_stuff):
my_string = ""
total = 0
for thing in made_up_stuff:
if type(thing) == str:
my_string += thing
elif (type(thing) == float or type(thing) == int) and thing != str:
total += thing
# Unindent the next two lines so they are not inside the for loop
total = str(total)
my_string += str(total)
return my_string
I hope this helps - let me know if it works!