Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial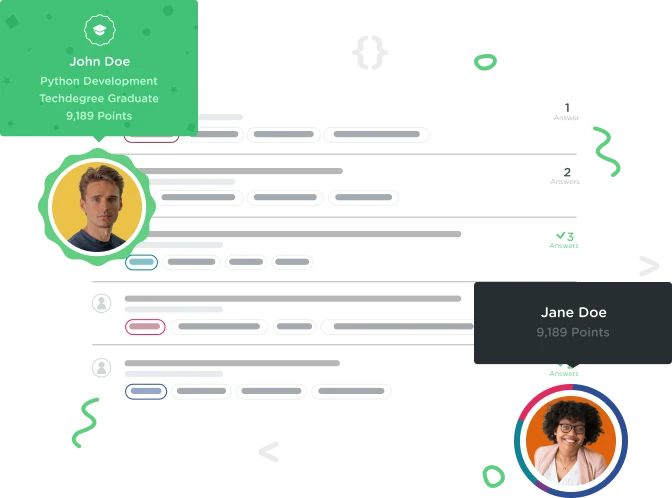

thomas2017
1,342 PointsPython, Collection, Set Math task 1 of 2 *Alternative way using for loop and if statements
I was wondering my thinking process would work? I know I was suppose to use intersection to solve this but this was the best that I could muster up before I looked up for the answer. How the heck were we suppose to solve this using the material from the video? When I ran it I got an error of 'dict' object has no attribute 'append'. Can someone please let me know why I got that error.
This was the answer someone else posted.
def covers(arg):
hold = []
for key, value in COURSES.items():
if value & arg:
hold.append(key)
return hold
covers({'Ruby'})
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(topics)
#x,y,z
set1 = {}
for item in topics:
if item in "Python Basics":
if "Python Basics" not in set1:
set1.append(item)
else:
continue
elif item in "Java Basics":
if "Java Basics" not in set1:
set1.append(item)
else:
continue
elif item in "PHP Basics":
if "PHP Basics" not in set1:
set1.append(item)
else:
continue
else:
if "Ruby Basics" not in set1:
set1.append(item)
else:
continue
return set1
1 Answer
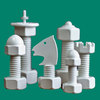
Steven Parker
231,269 PointsThe other person's answer appends to a variable named "hold" which was initialized with "hold = []
" notice brackets. This makes it an (empty) list, and a list is what the challenge asked for as a result anyway.
In your code you are attempting to append to "set1" which was initialized with "set1 = {}
" notice braces. That makes it a dict instead of a list, and as you discovered, a dict has no "append" method.
So that explains that particular error. But also, tests like this probably don't do what you were expecting:
if item in "Python Basics":
This does not check the item against the values associated with the dictionary key "Python Basics". What it actually does instead is check to see if the item is contained in the literal string "Python Basics". So if item
contains "sic" the result would be True, but if item
contains "arrays" the result would be False.
thomas2017
1,342 Pointsthomas2017
1,342 PointsHi Steven thanks for the explanation. I was wondering what do you mean "sic" ? " So if item contains "sic" the result would be True, but if item contains "arrays" the result would be False."
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe string "sic" would match part of "Python Basics" (note underlined substring). My point was that the code line is looking for matches in the words "Python Basics", not in the dictionary values associated with that key.